
What is a State variable?
When a State value changes, SwiftUI updates the parts of the view hierarchy that depend on the value. State variables form the foundation of creating interactive SwiftUI experiences.
Declaring a State variable
Declaring a State variable is similar to declaring a traditional variable, the main difference is the @State
property wrapper prepended to the declaration.
When creating a State variable, SwiftUI will handle updates to the variable and re-render the views as needed, when the State is updated.
Declaring state as private prevents the value from being set by the view's initializer, which can cause conflicts with SwiftUI's state management.
Setting a State variable
You can set a State variable in your logical code such as a Button's action.
In this example, when the "Eat a cookie" Button is tapped, the Text will re-render its contents and show the updated value of the counter
State variable.
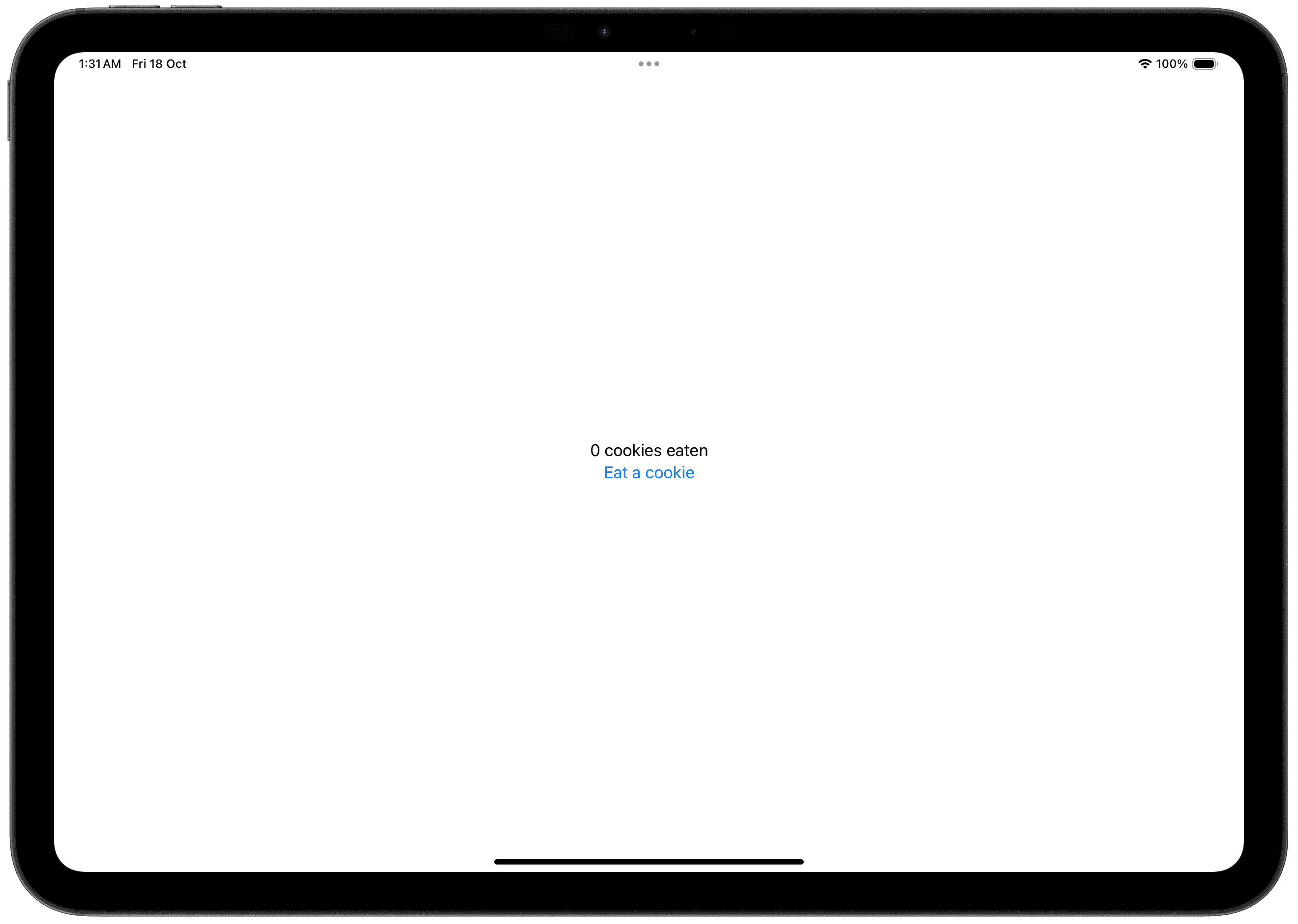
Observing State changes
You can use the .onChange
modifier to observe for changes in a State variable. This allows you to access both the newly-set value and the previous value.