Getting Started
Creating a Button
To create a button, you need to supply it with a Label—the content of the button, and an Action—the code executed when the button is tapped.
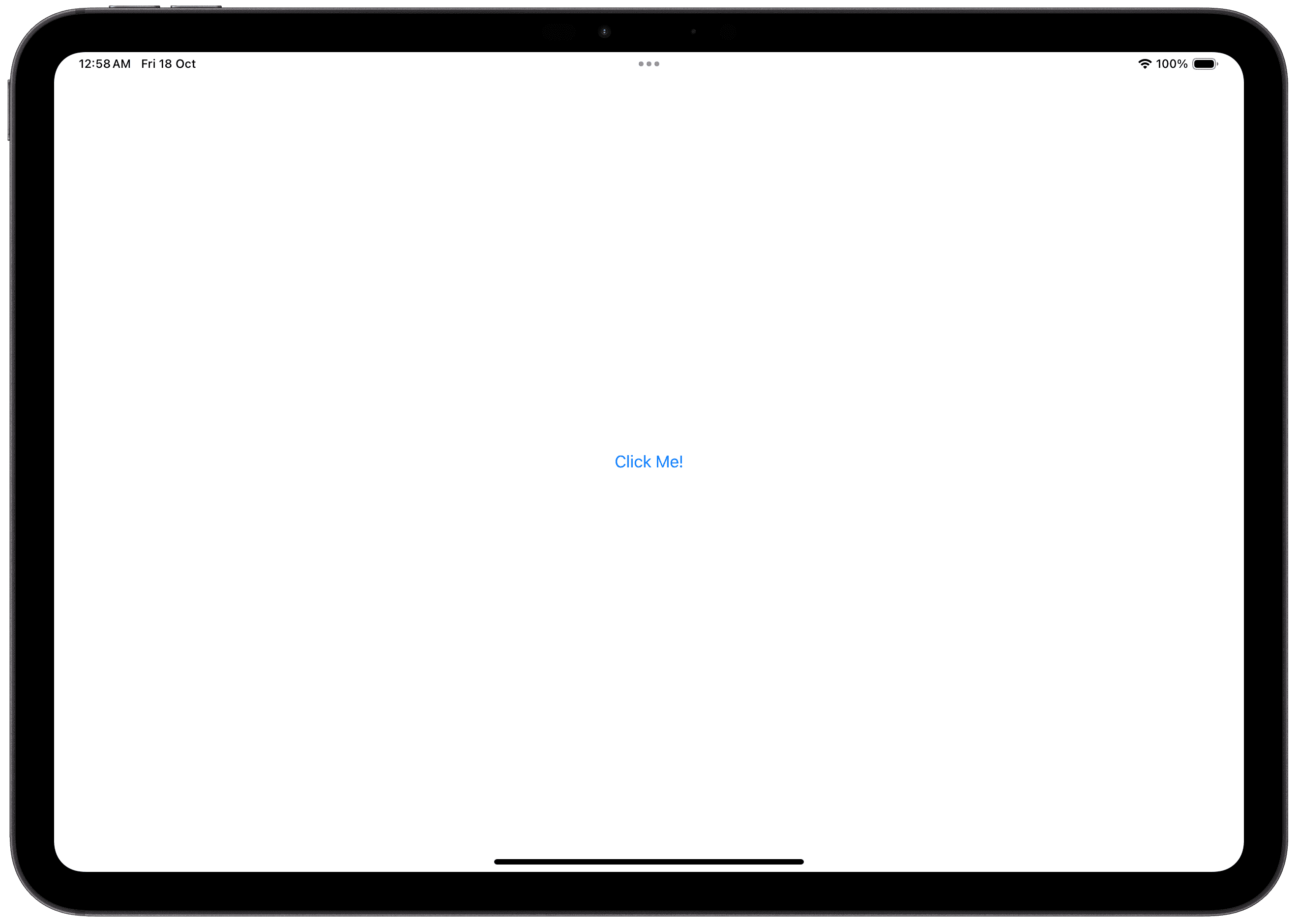
SwiftUI provides several different ways to create a Button. Each approach provides a different way for you to customize the Action and Label, providing differing amounts of customizability.
Text Label
This is the simplest implementation of a button. It allows you to quickly create a simple button, but does not afford much customization.
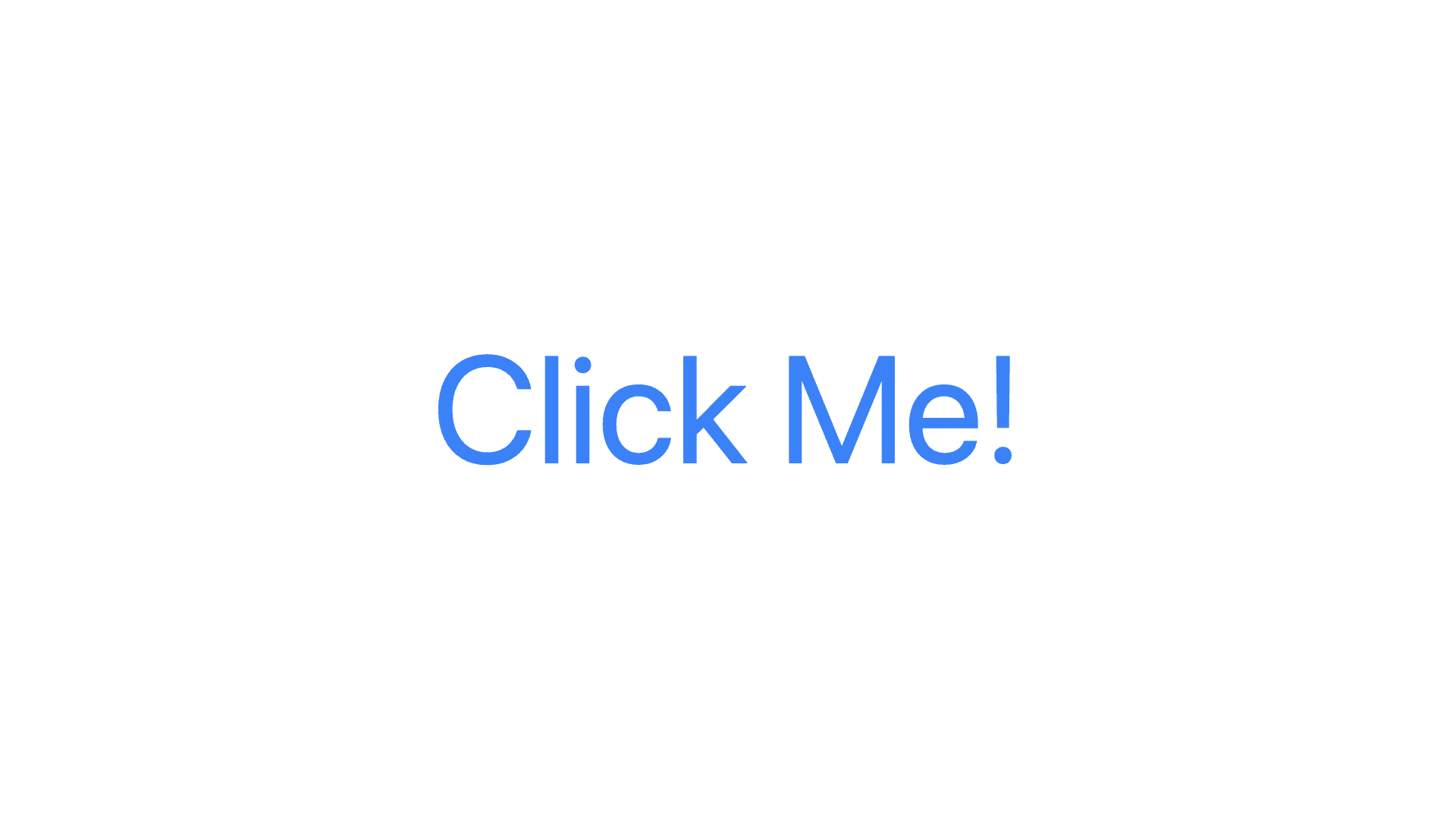
Custom Label
You can supply a custom label using this Button initializer. This implementation gives you more control over the appearance of the button.
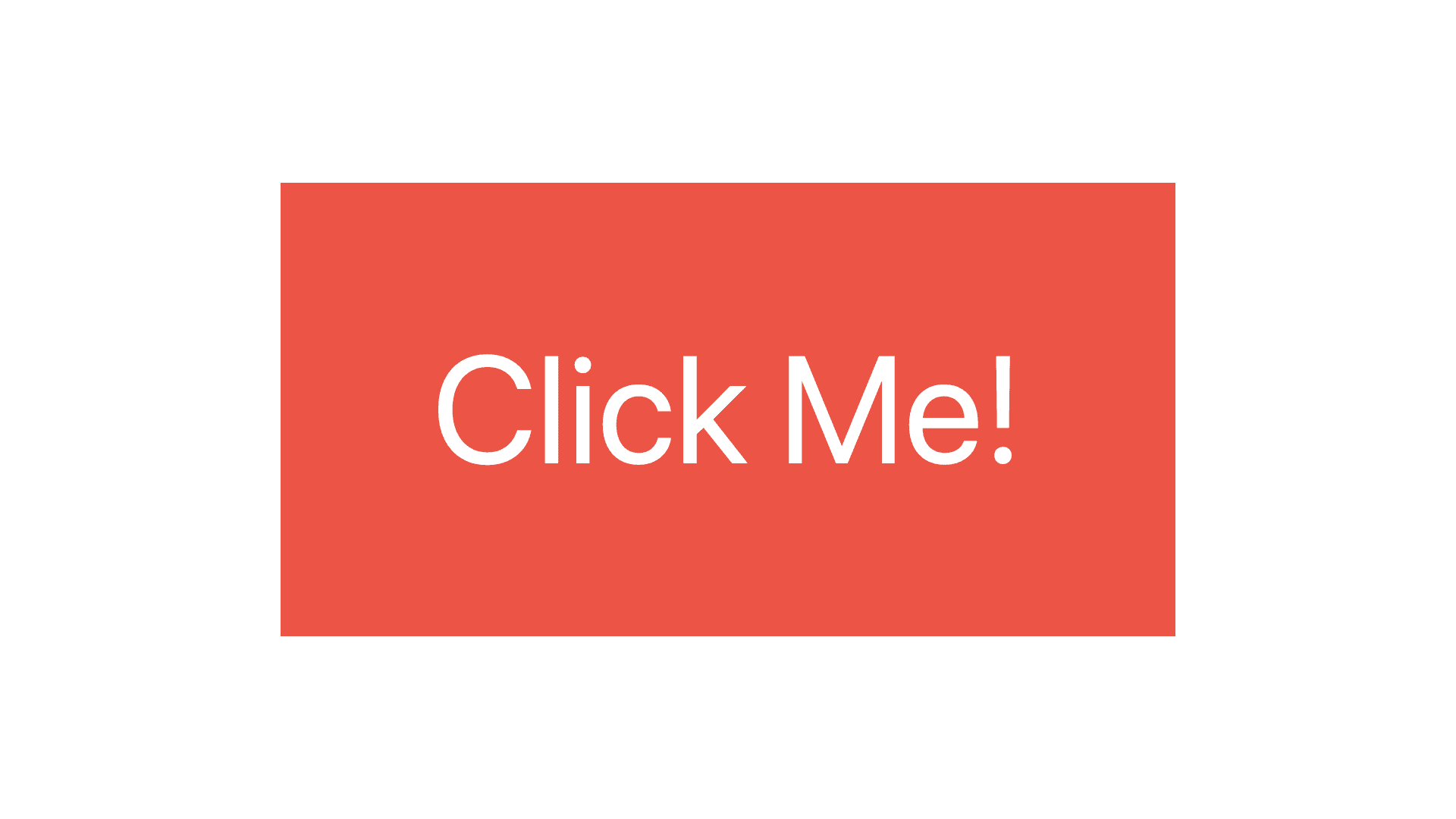
Image Labels
You can also supply an image or SF Symbol to quickly create a customized button with an image.
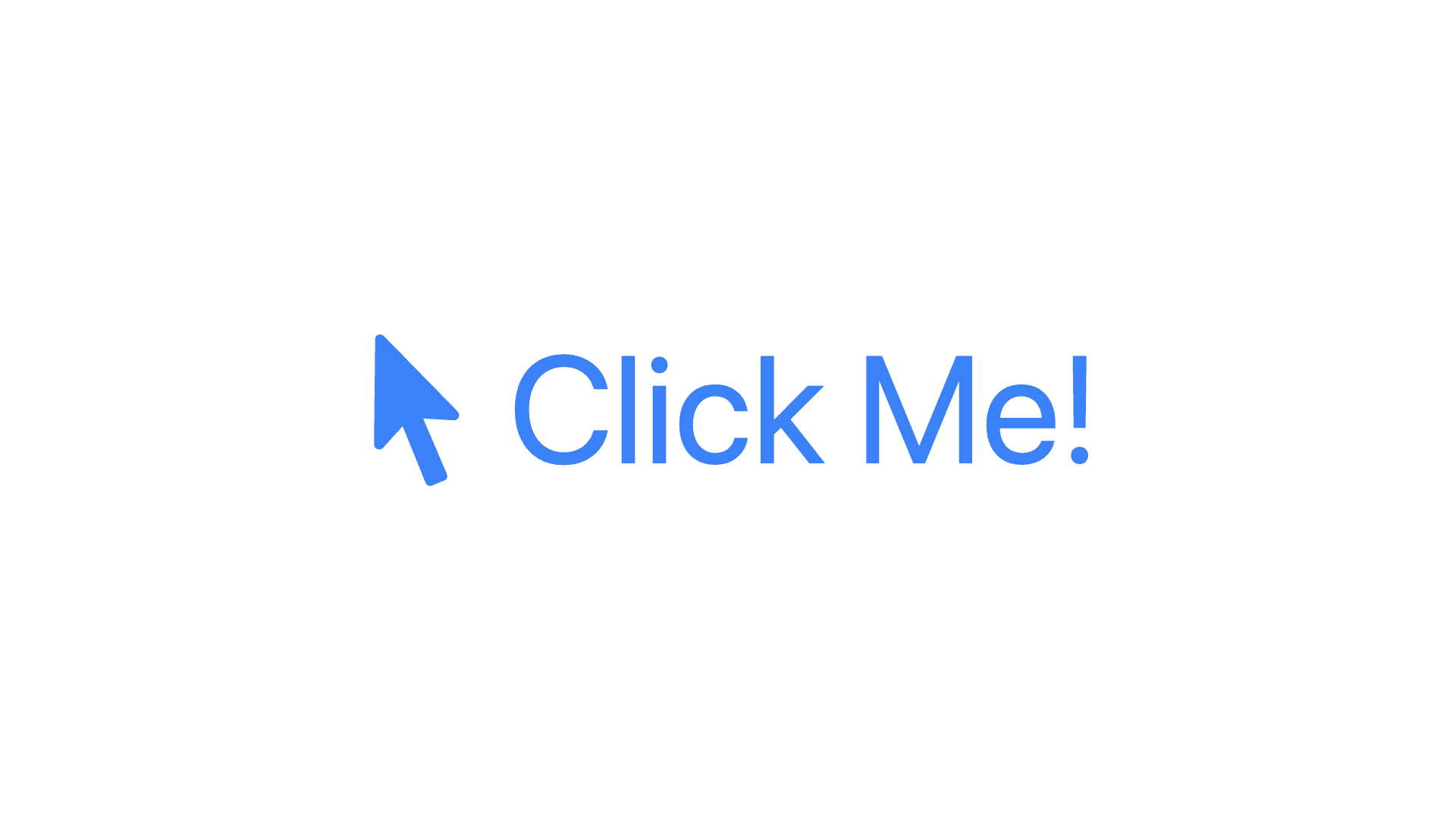
View Builder & Logical Code
SwiftUI provides various spaces for View Builder and Logical Code.
View Builder code is code that describes how your SwiftUI view looks like. This is where you can use your
Text
,Button
, and other views.Logical code is traditional Swift code like if-else statements, setting variables, loops, etc.
Going Further
Button Styles
You can customize a button's appearance with the buttonStyle
modifier.
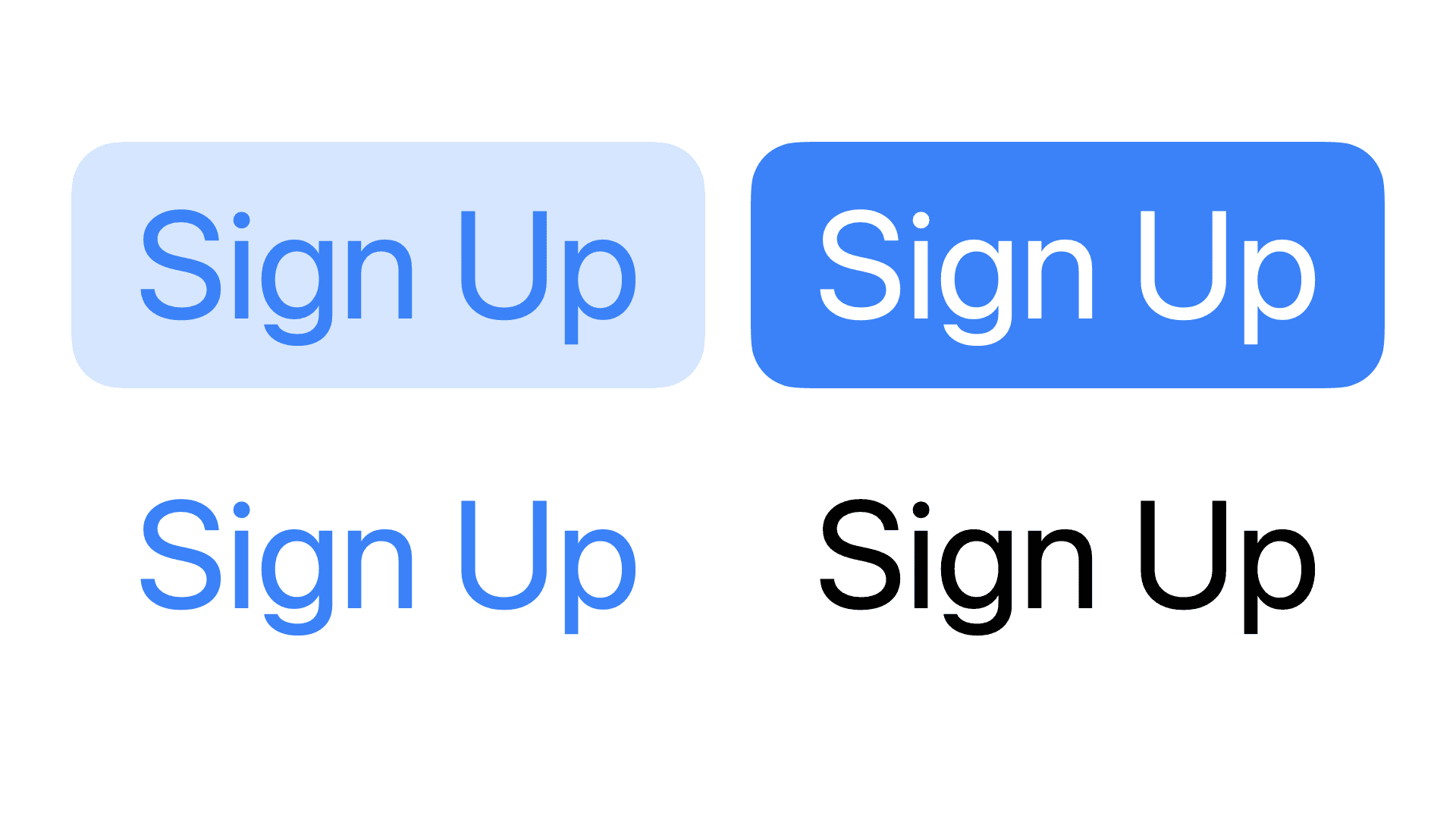
Cascading Styles
You can apply a style to a parent view and have its child views reflect the style.
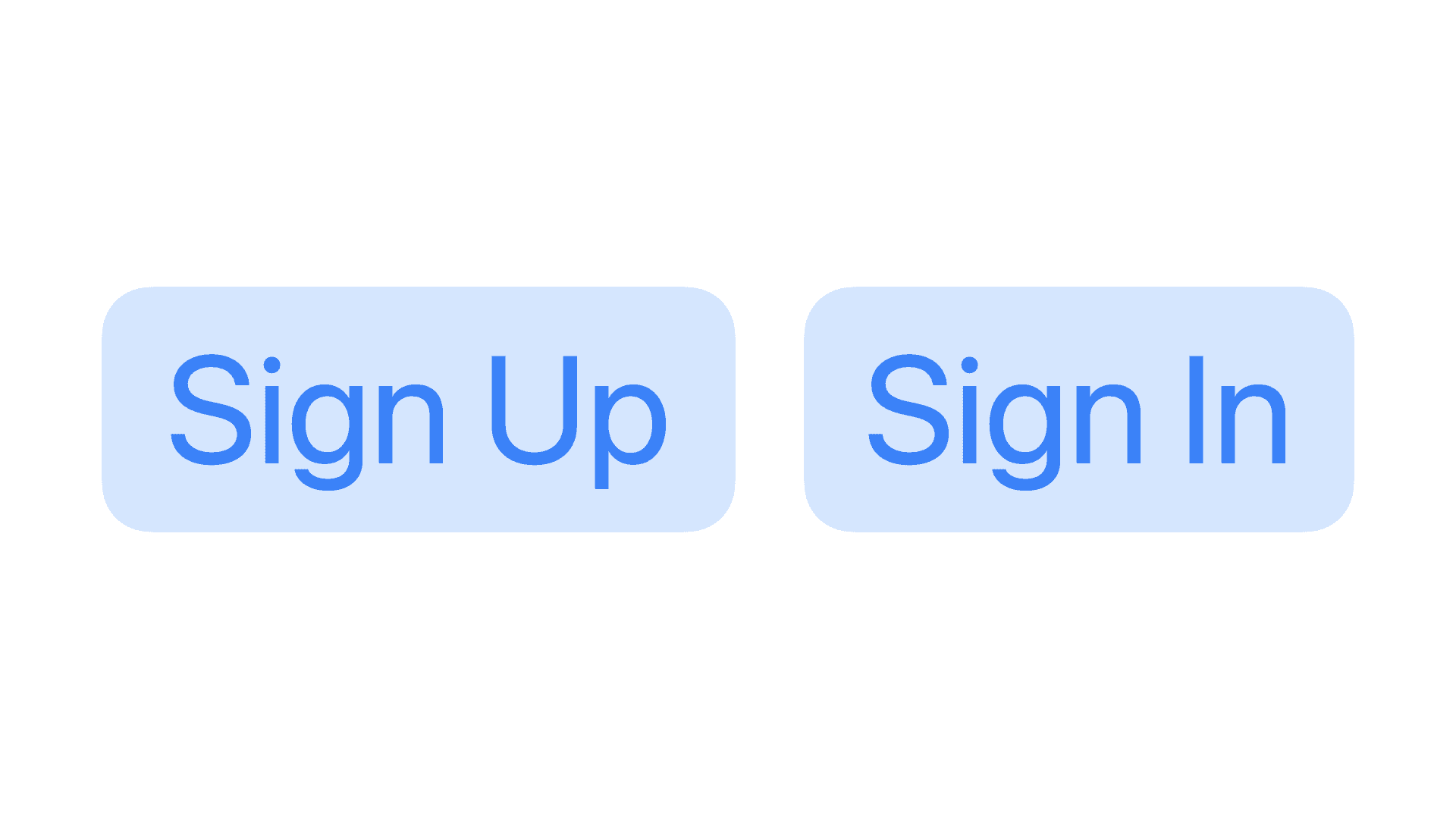
Button Roles
You can supply your button with the role
parameter to characterize your Button's purpose. For example, for an irreversible action like deleting data, you may choose to assign it a .destructive
role. Refer to the Human Interface Guidelines for guidance on the various button roles.
By default, a .destructive
role will give the icon a red tint to draw attention to the action.
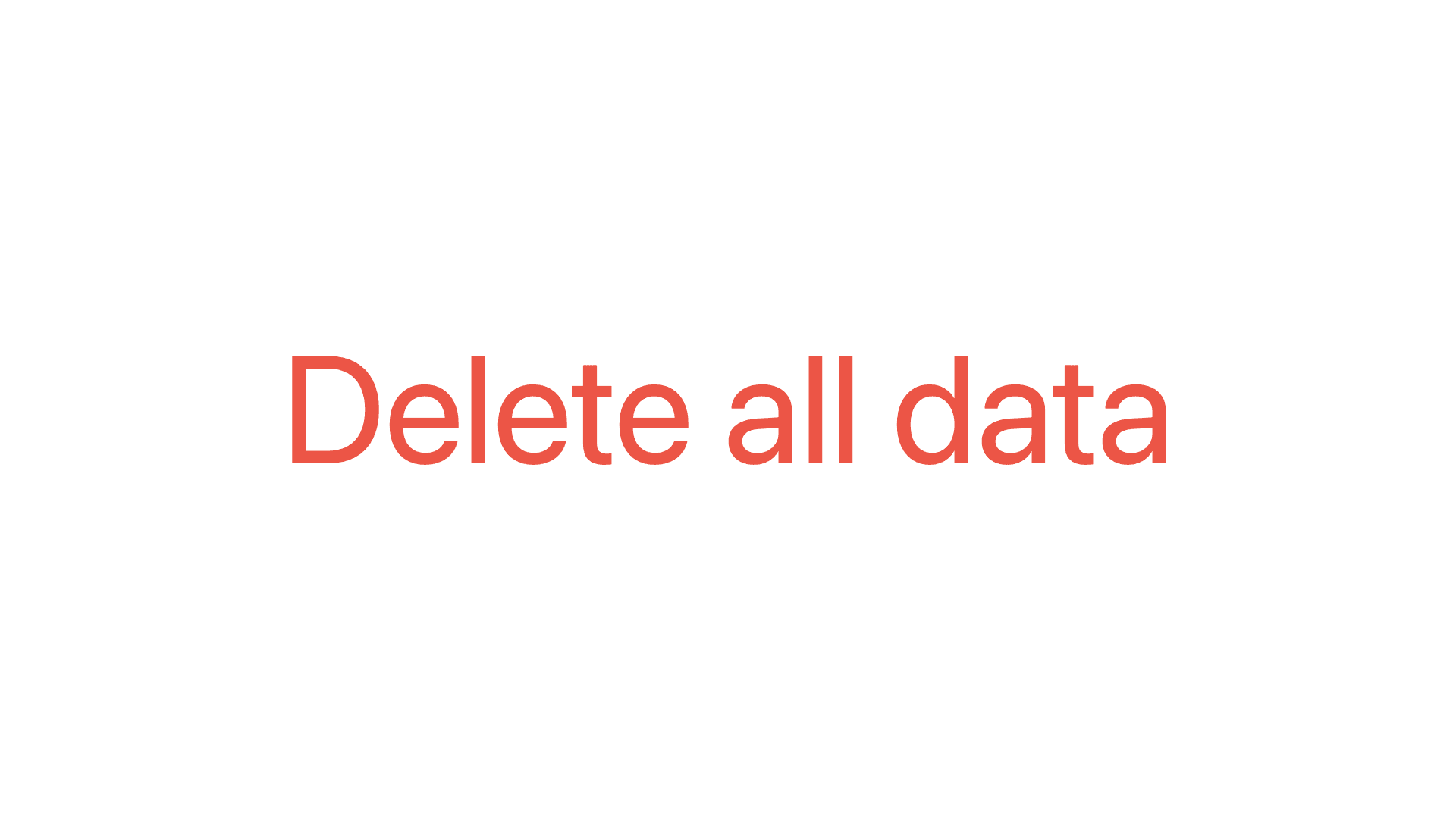
Refactoring Actions
You can clean up your code by refactoring actions into their own functions.
This works with all Action closures, giving you a way to quickly and easily clean up complex views.
In These Collections
This article can also be found in these collections.