Creating a Toggle
To create a toggle, you will need to supply it with a Binding boolean and a Label—a companion label to describe the toggle's content.
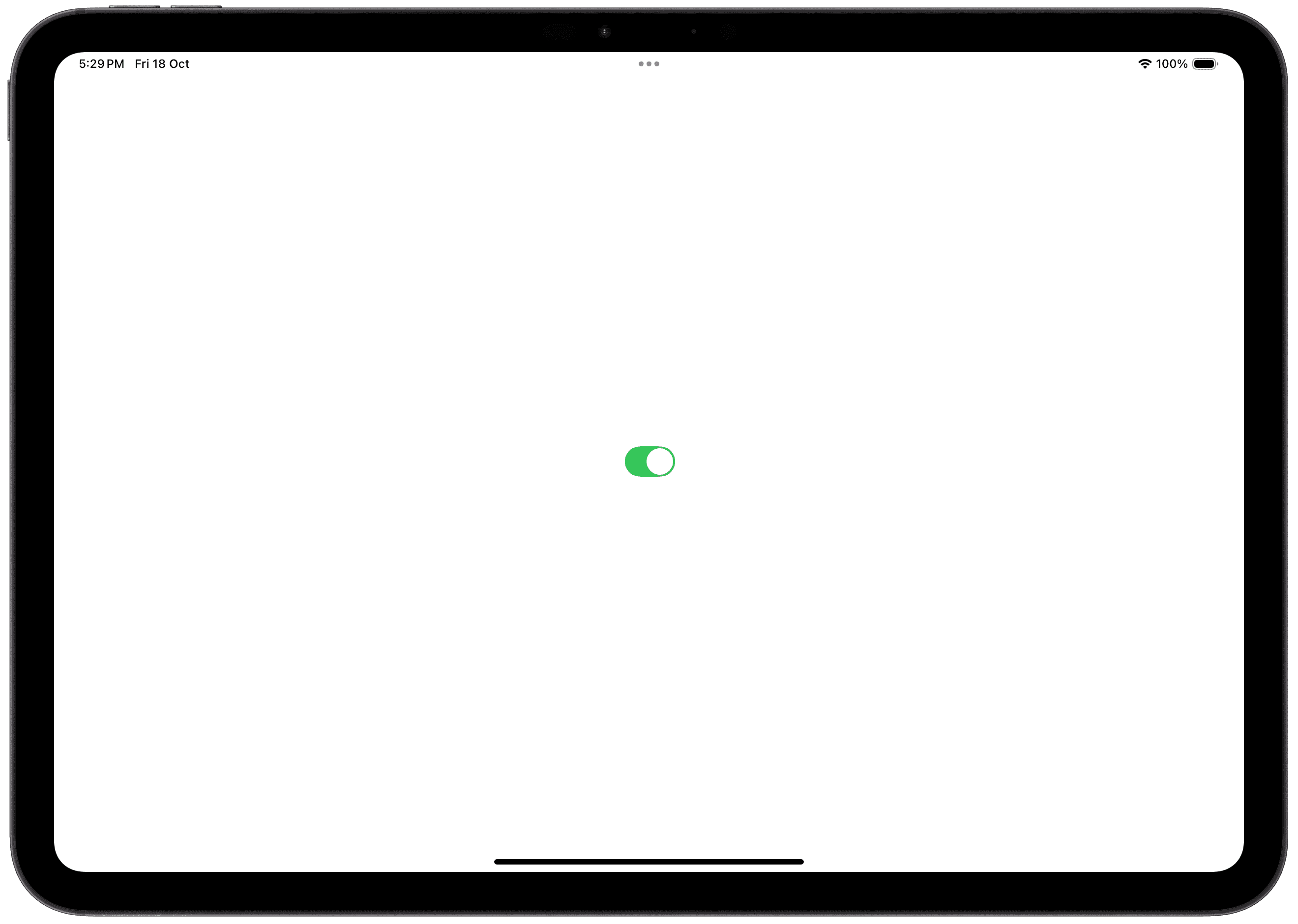
Basic Toggles
Text Labels
The simplest way to create a toggle with a simple text label.
Note that, in this style, by default, tries to take up as much space as possible. It will attempt to stretch to fill the entire width of the parent view.
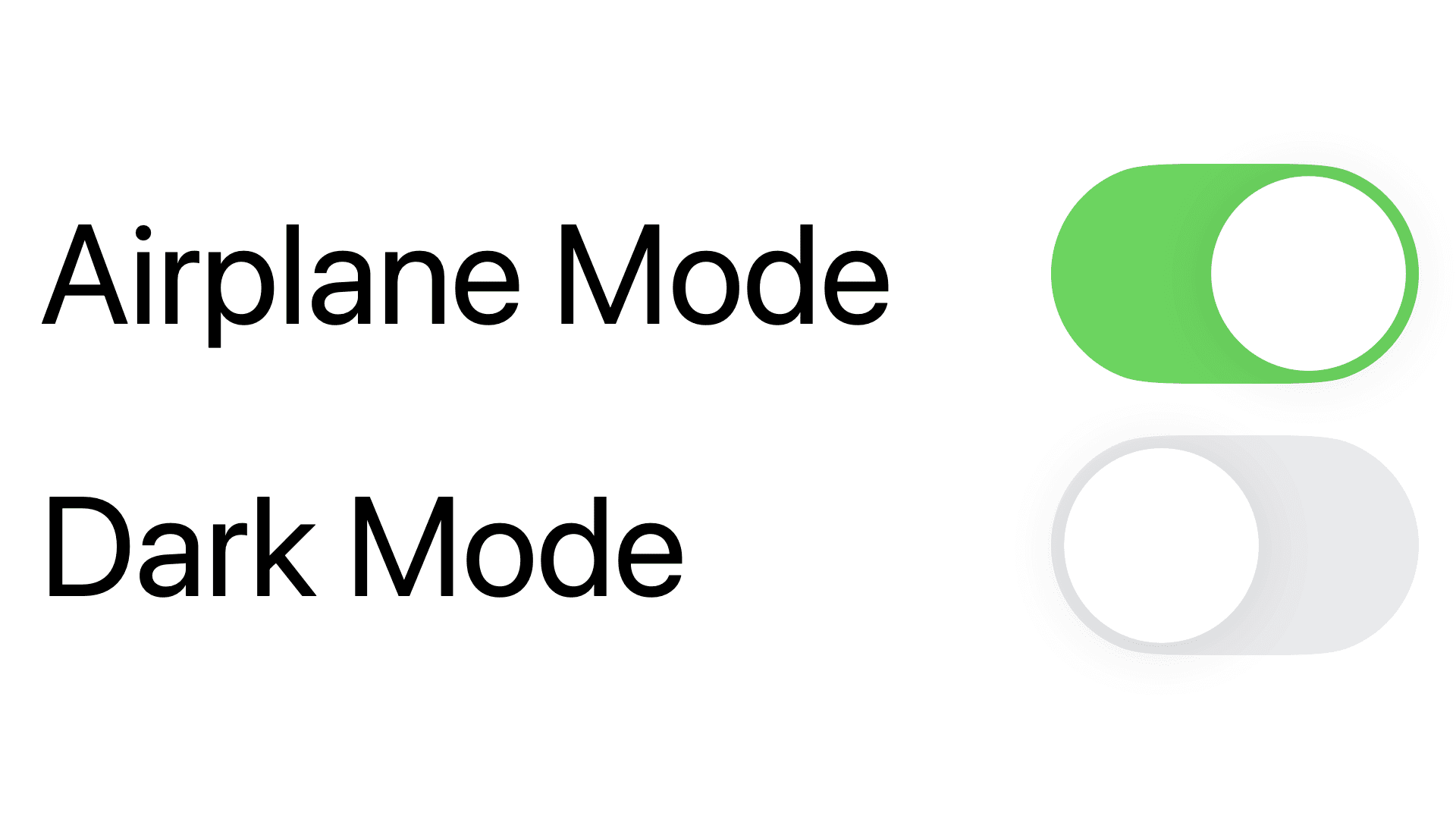
Text and Image Labels
Like Buttons, you can set an image in the Toggle's label.
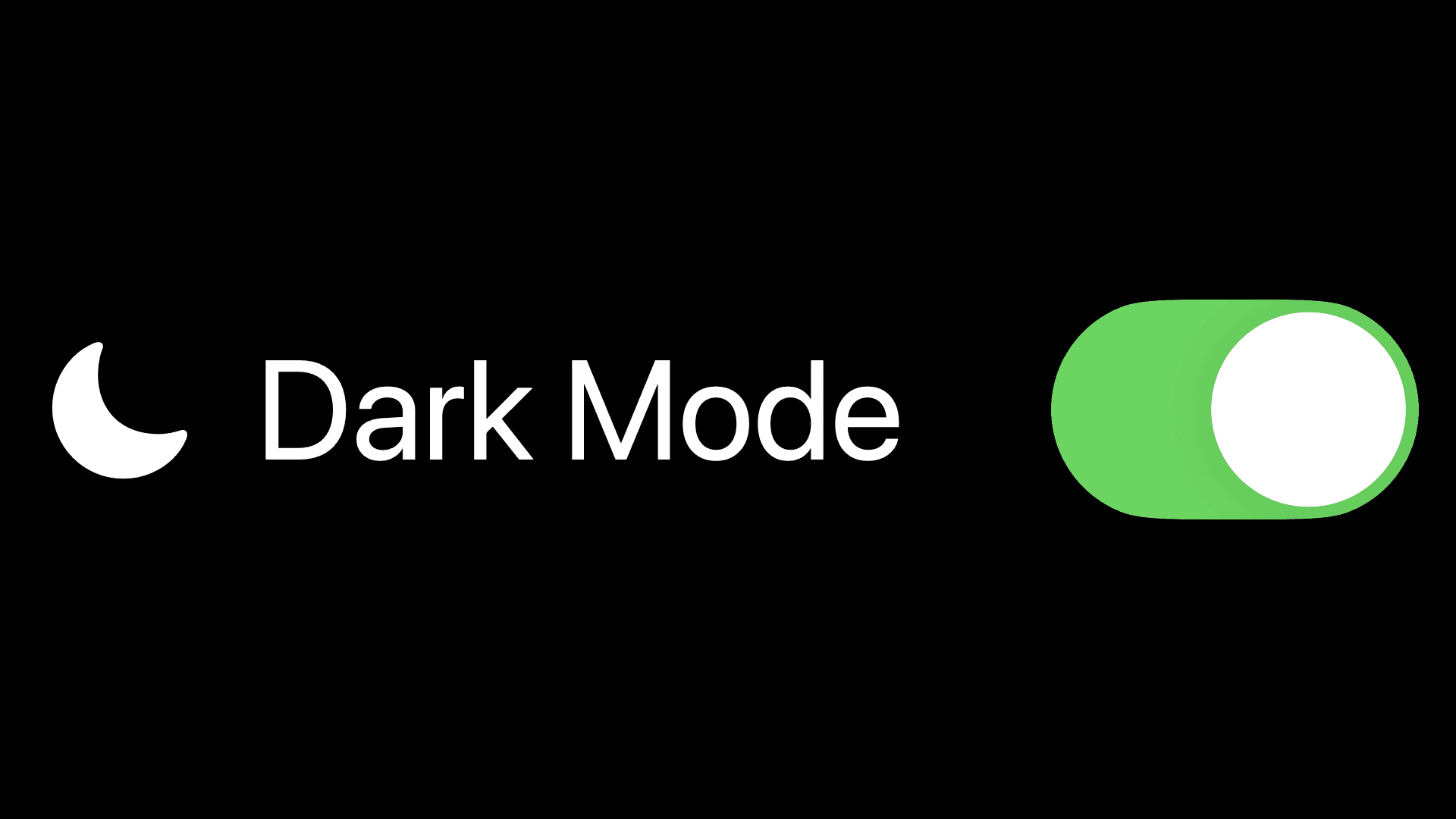
Custom Labels
You can also supply a custom view as the toggle's label.
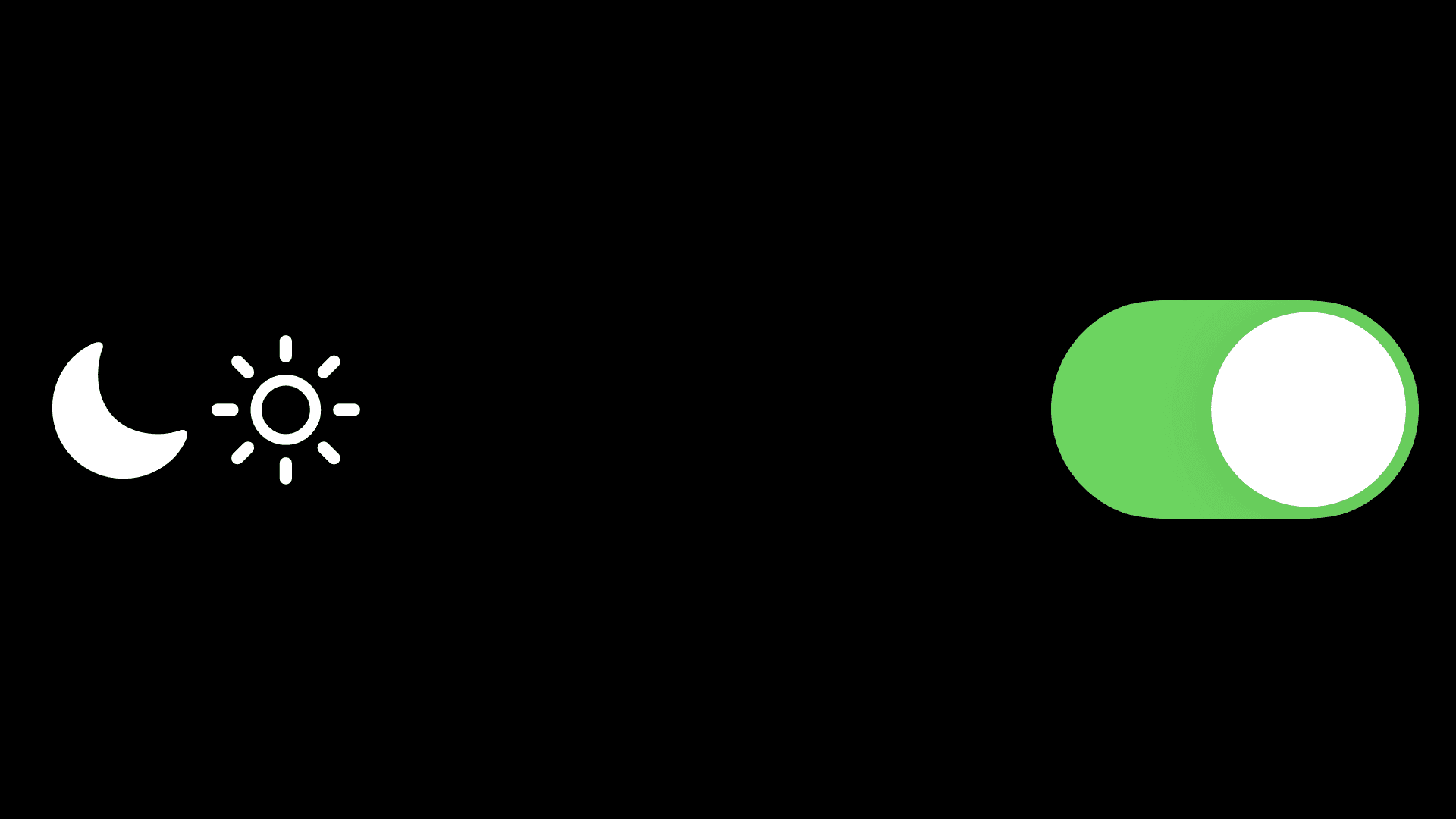
Toggles with Collections
Toggle multiple boolean properties across a Binding collection, like an array. This can be used to mark all messages as read, or to disable all alarms.
To do this, you will need a struct with a boolean property to toggle. In the following example of an email app, a struct of Mail
might have an isRead
property.
If any of the mail is unread, it will mark all as read. If all mail is read, it will mark all of them as unread.
Text Labels
You can use a similar initializer as the basic toggles, passing in an additional sources
parameter with a Binding collection of times. In the example, the Binding collection is incomingMail
, an array of Mail
.
The \.isRead
syntax is called a Key Path. It points to the property that should be toggled in the Mail
struct.
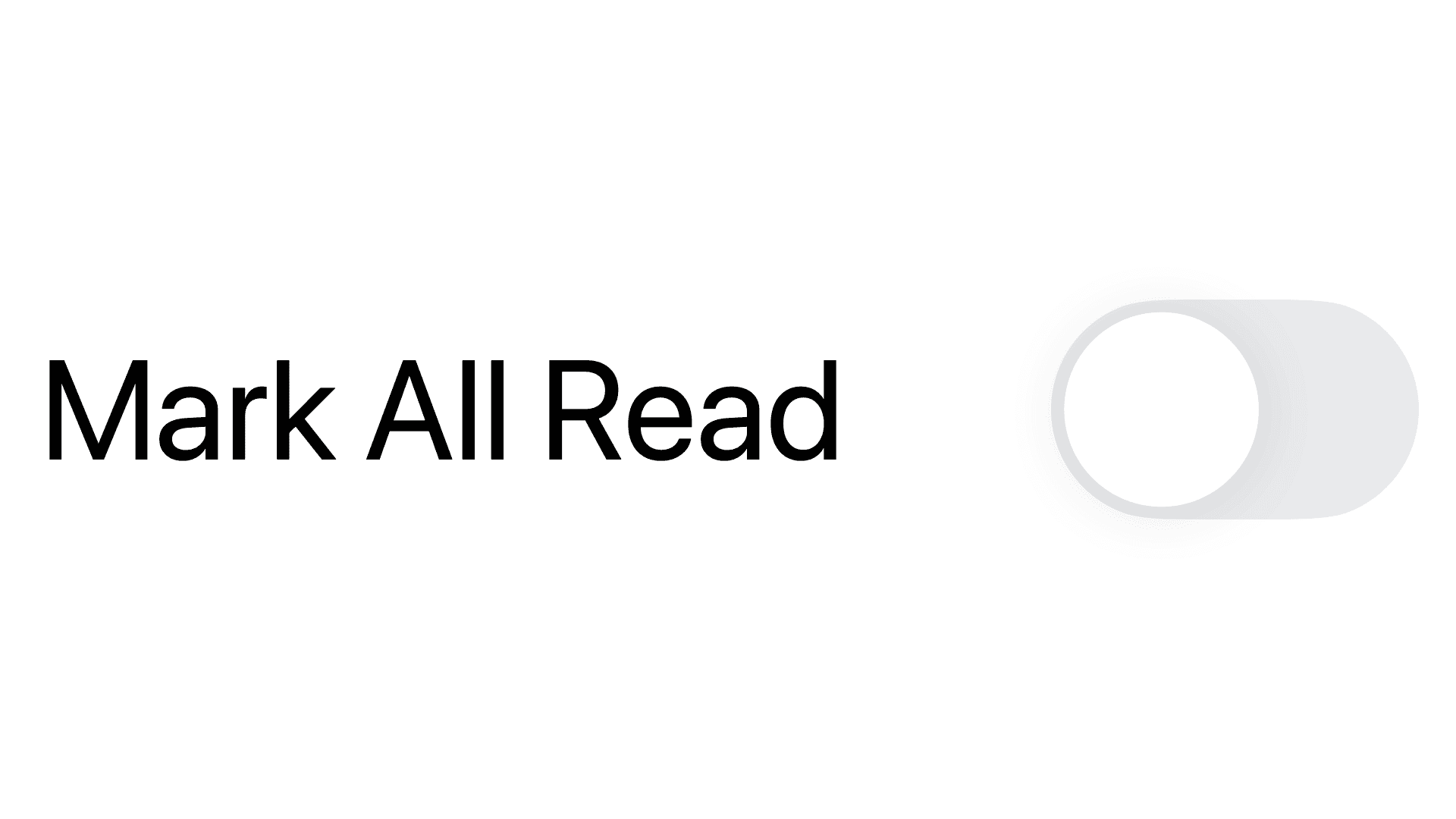
Text and Image Labels
Similarly, you can supply the systemImage
or image
parameter to add an image into the Toggle's label.
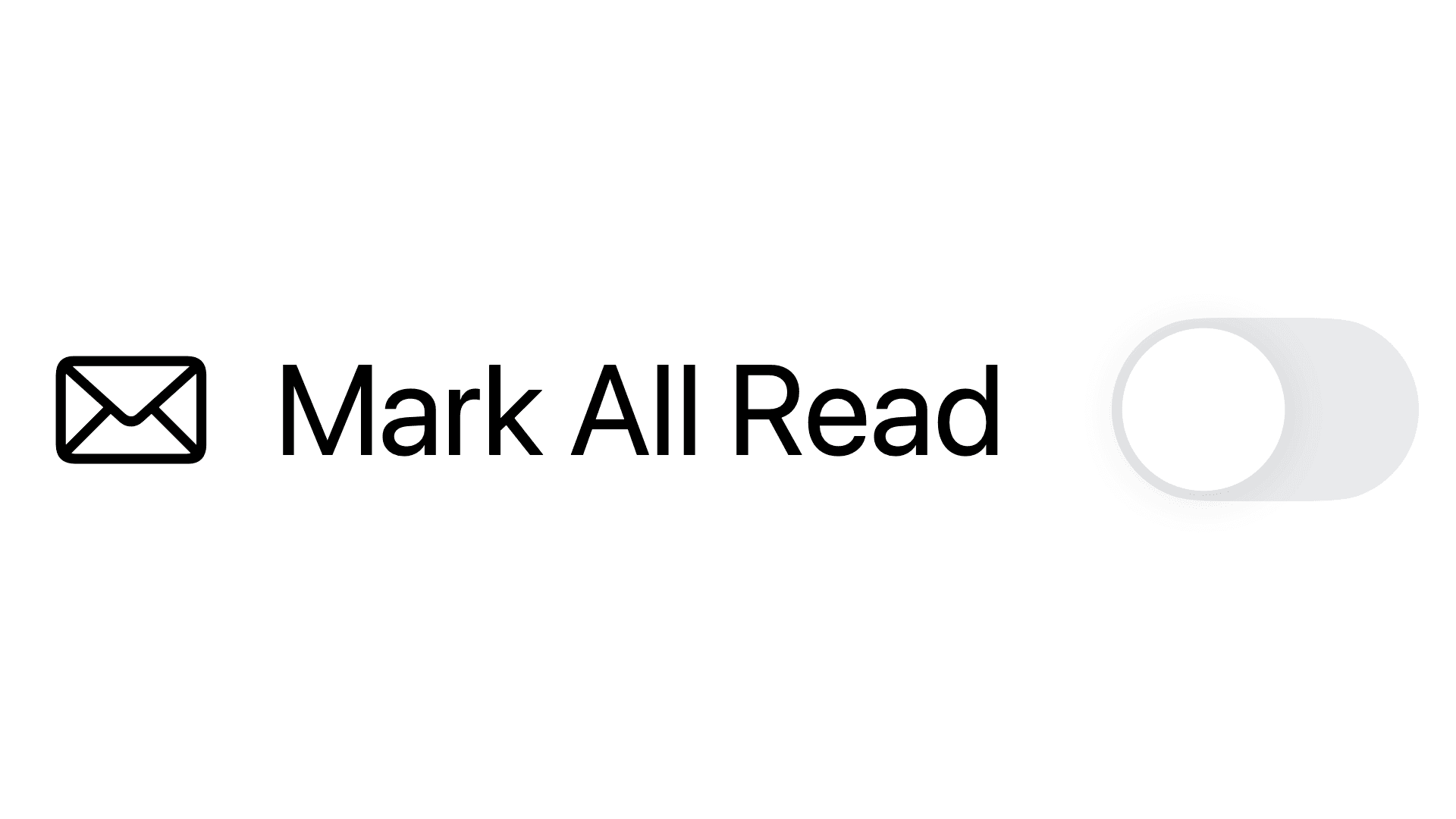
Custom Labels
You can also use a custom label for the toggle.

Styling Toggles
Toggle Styles
By default, toggles use a the .switch
style to create the familiar rounded switch, however, like button styles, you can customize this as well.
Switch Style
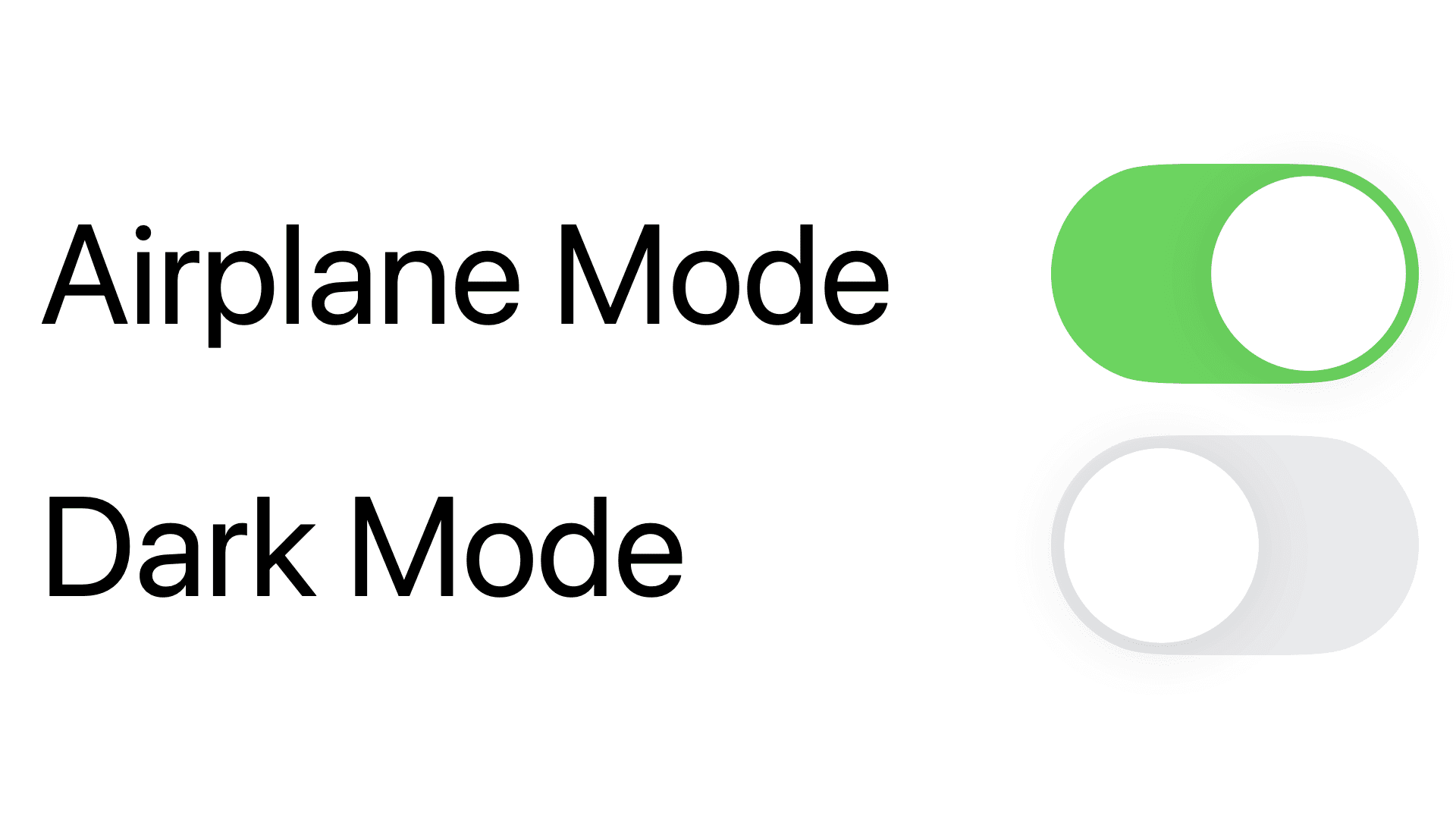
Button Style
The button style gives the toggle a bordered
button style when it is on and a borderless
style when off.
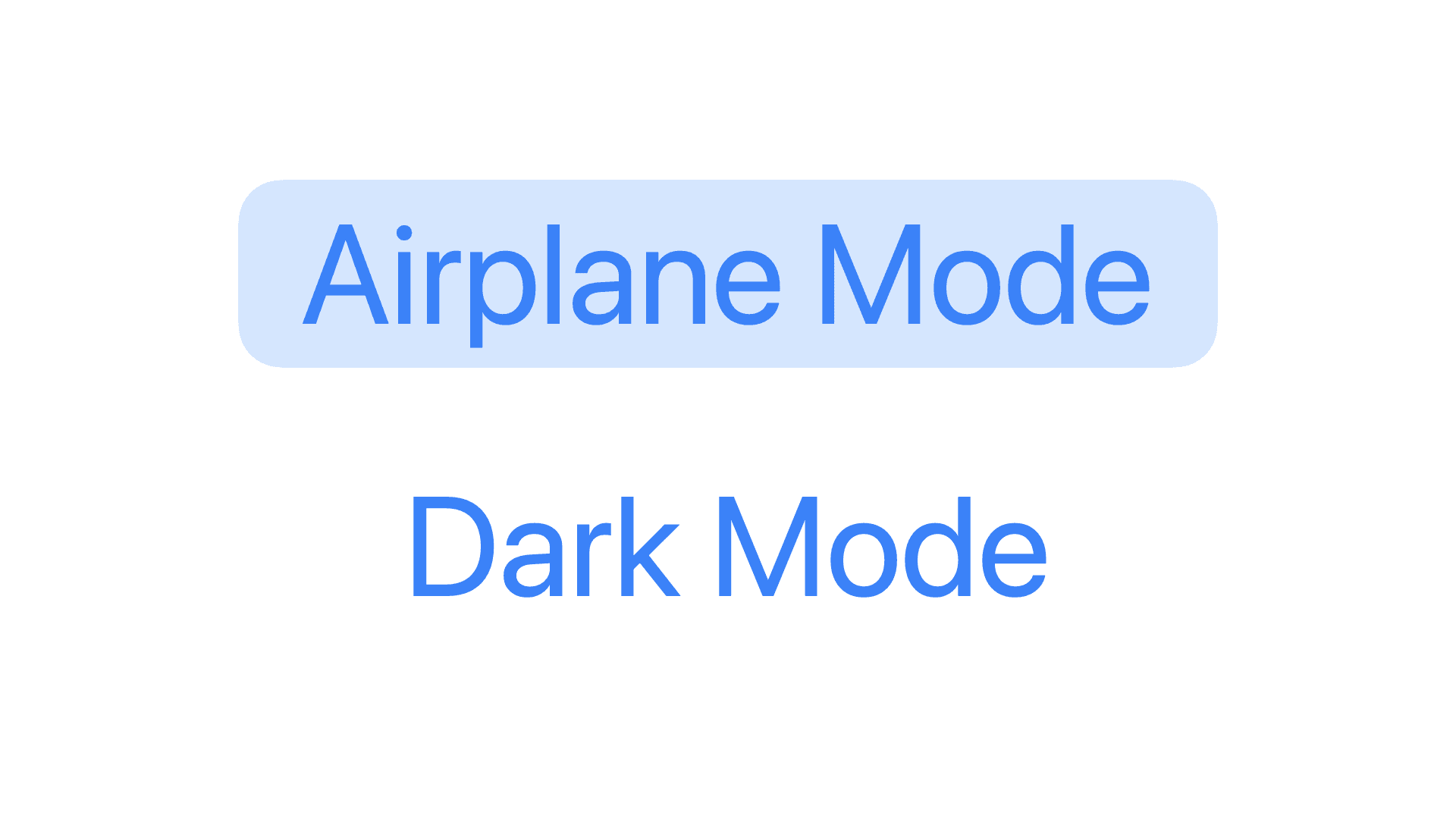
Hiding Labels
If you prefer not to have the label in your toggle, you can use the labelsHidden
modifier to remove it.
It is best practice to still use an appropriate text label for your toggle, even if it might not be visible. This can help with assistive technologies like VoiceOver.
Using the labelsHidden
modifier will not work for button-styled toggles.
In These Collections
This article can also be found in these collections.