Creating a Spacer
A Spacer is used to add a flexible space between subviews in a stack view like a VStack or HStack.
Spacer in Horizontal Stacks
When in a HStack, it spreads the elements apart horizontally.
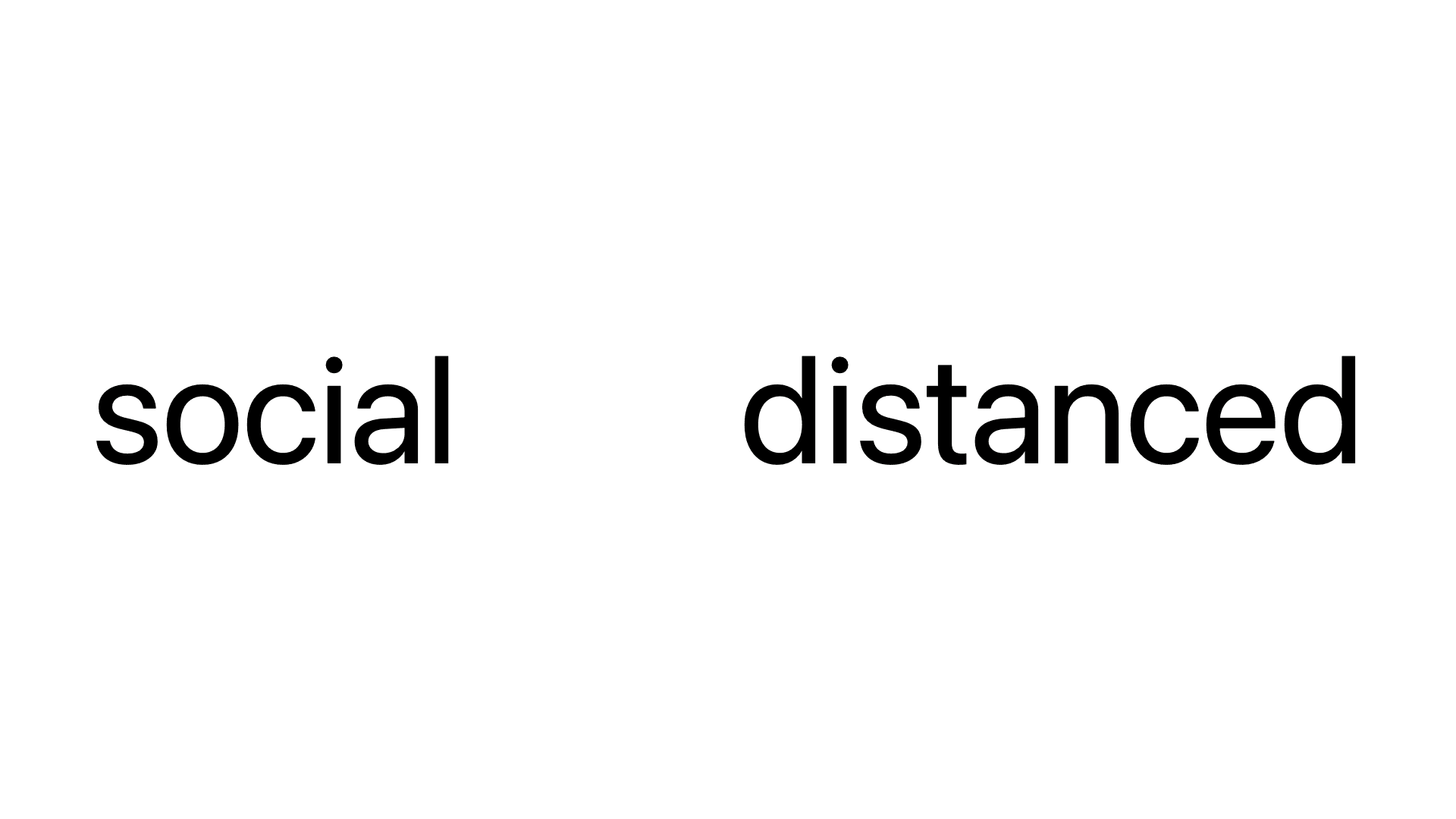
This can also be used to align elements together to the leading or trailing edge.
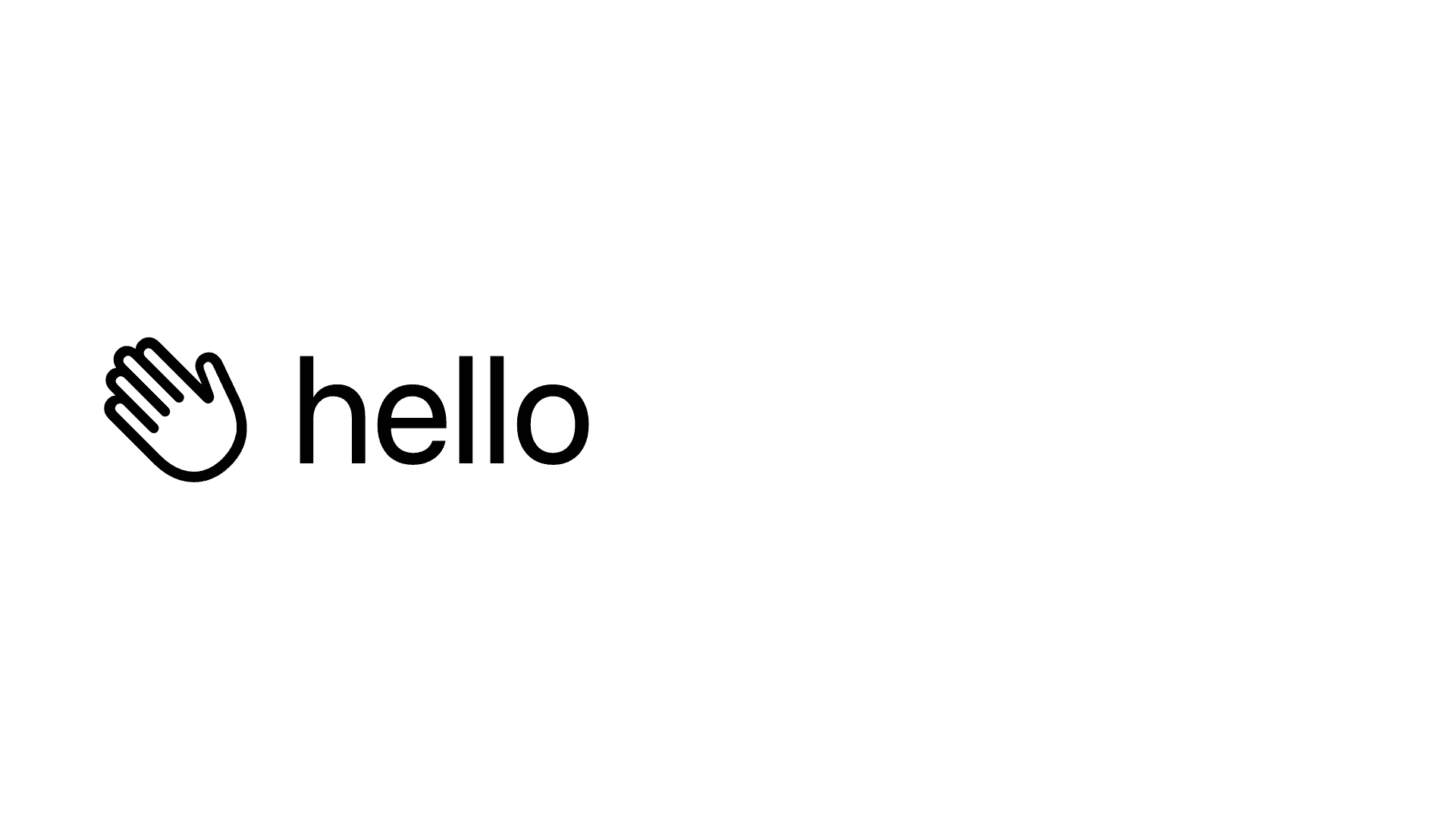
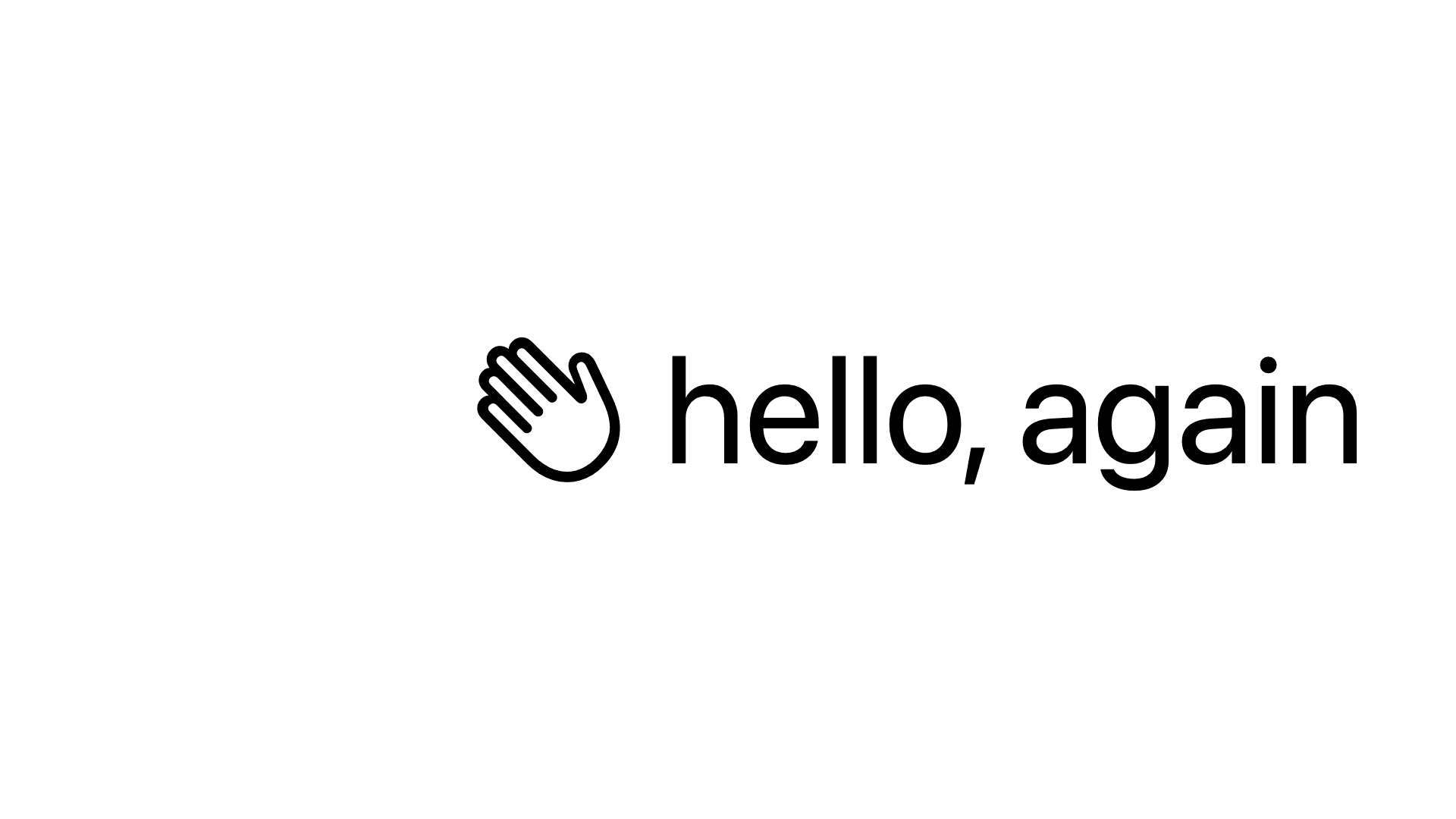
Spacer in Vertical Stacks
When in a VStack, the Spacer spreads elements apart vertically.
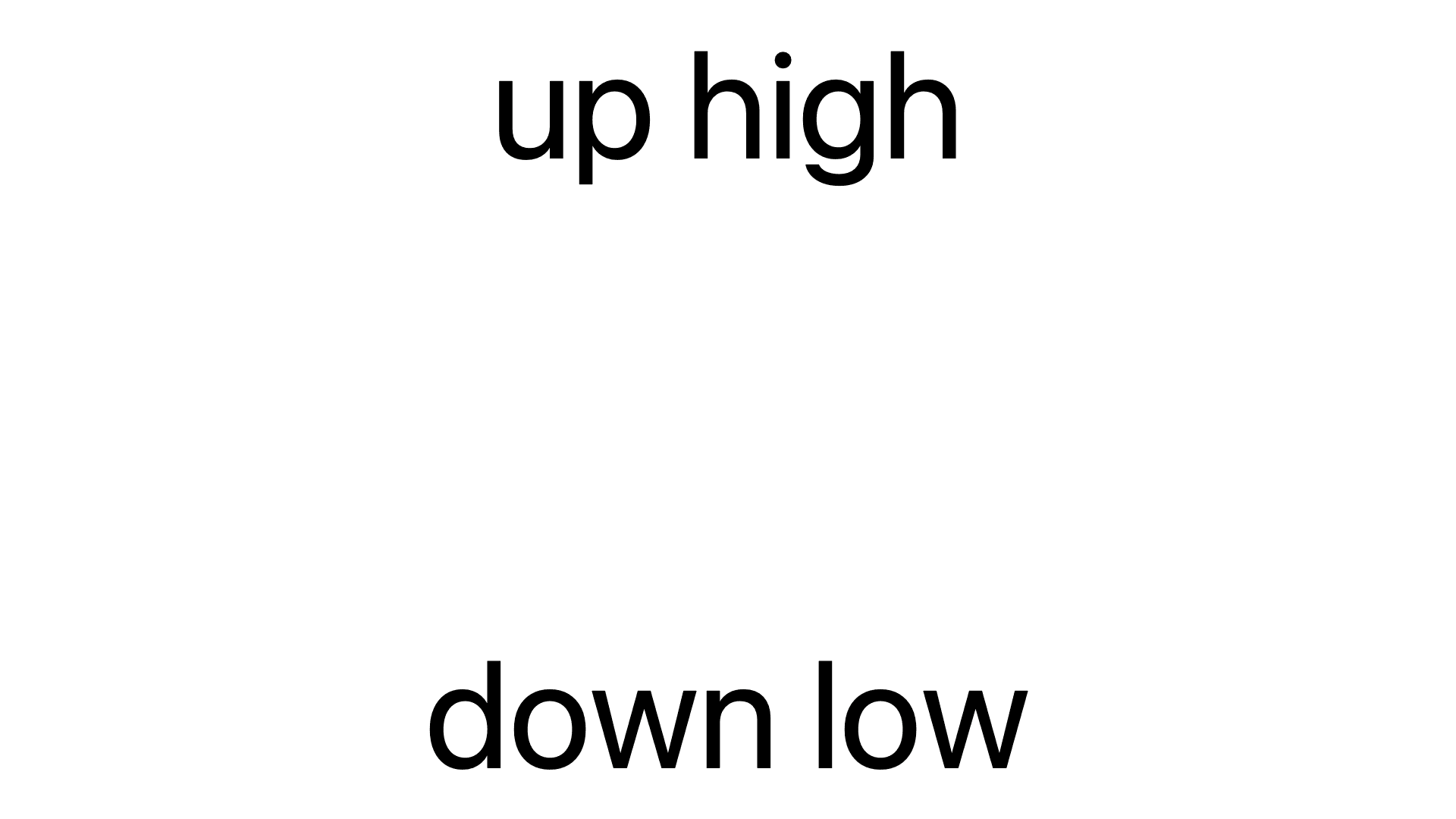
This can also be used to align to the top and bottom edges of the parent view.
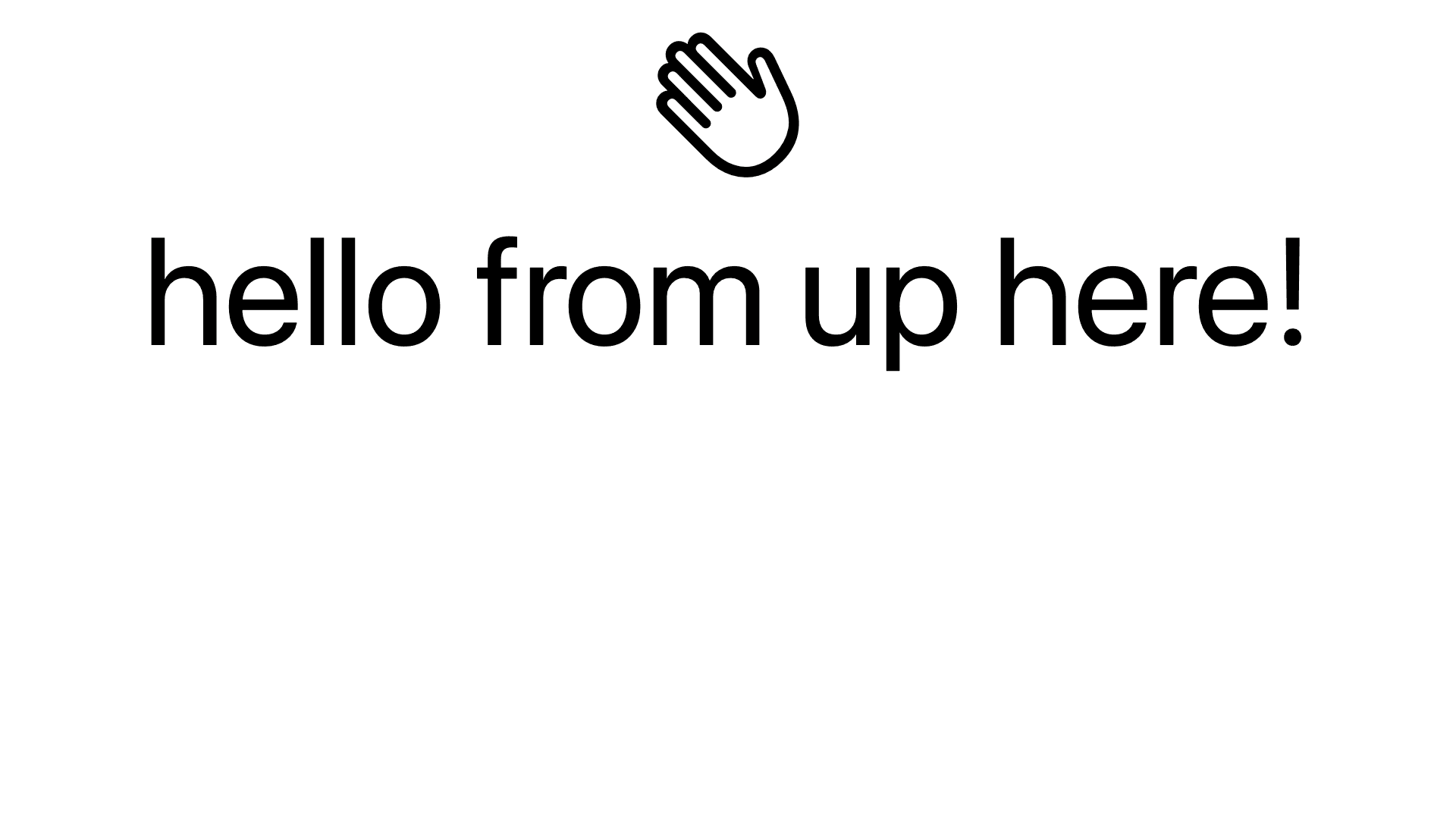
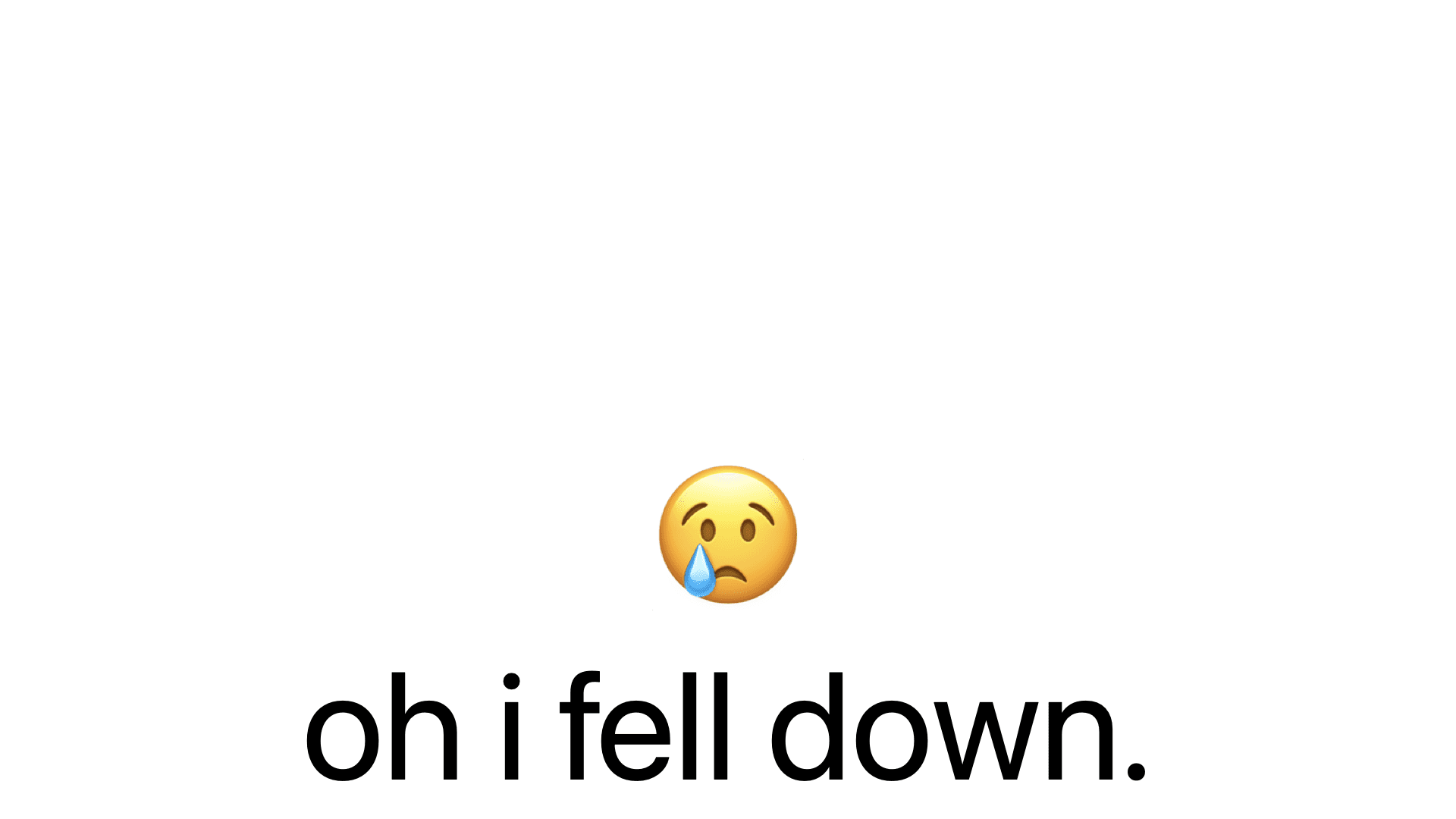
Using Multiple Spacers
You can also use multiple spacers to set different distances.
In the following example, the gap between the 🍎 and 🍋 is twice that of the gap between 🍌 and 🍎.

Setting a Minimum Length
You can also specify a minimum length for a Spacer, ensuring the Spacer does not fall below a certain size.
In These Collections
This article can also be found in these collections.