Creating Colors
Colors are used widely throughout SwiftUI to set text colors, background colors, and more.
Predefined Colors
SwiftUI has a set of predefined colors. These colors adapt automatically to dark and light mode.
In the following example, you can use Color to create a red text.
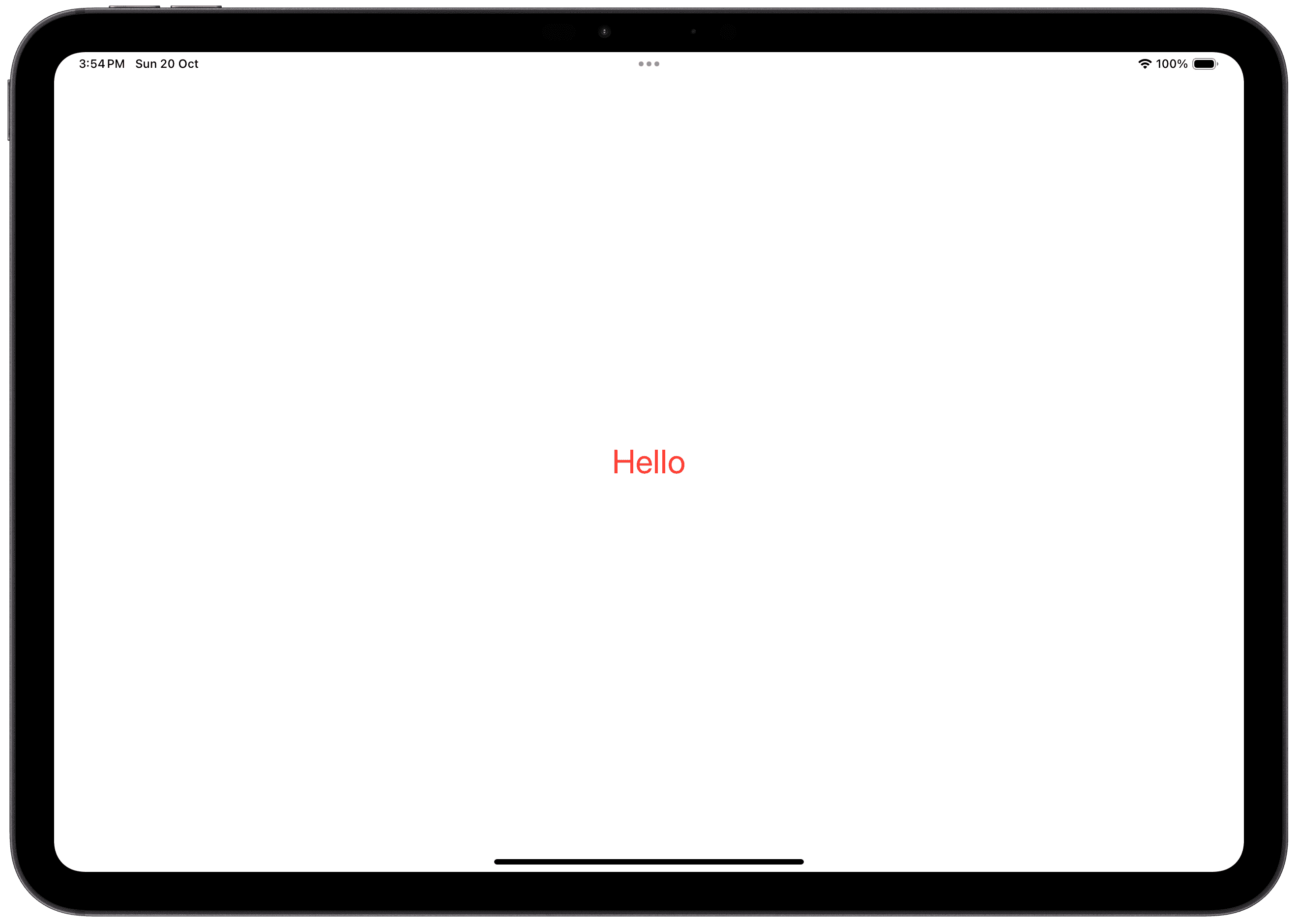
Or use Color to create a background.
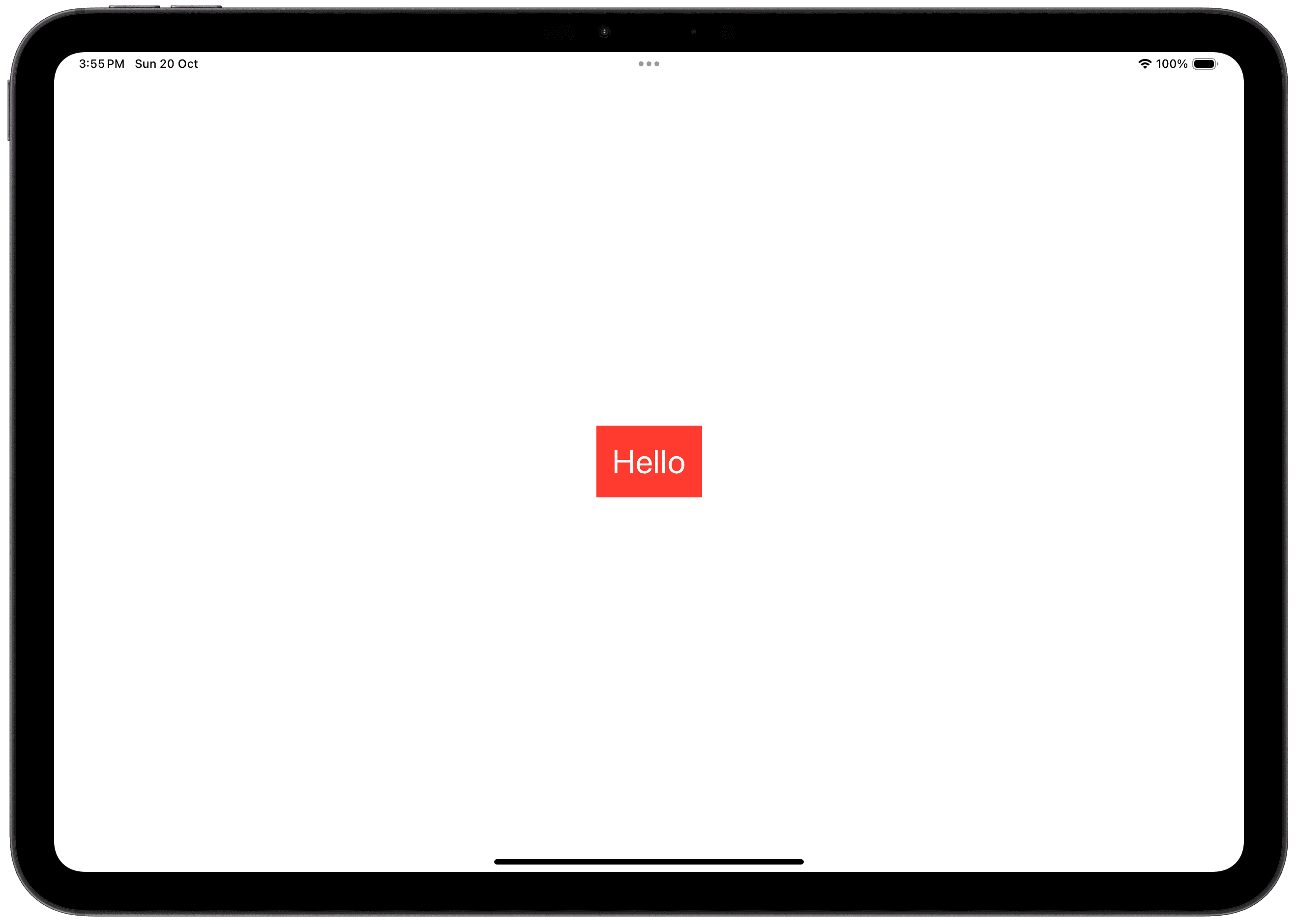
To use predefined colors, you can access it with Color.colorName
. Below are some examples of the colors. For a full list, refer to the Human Interface Guidelines.
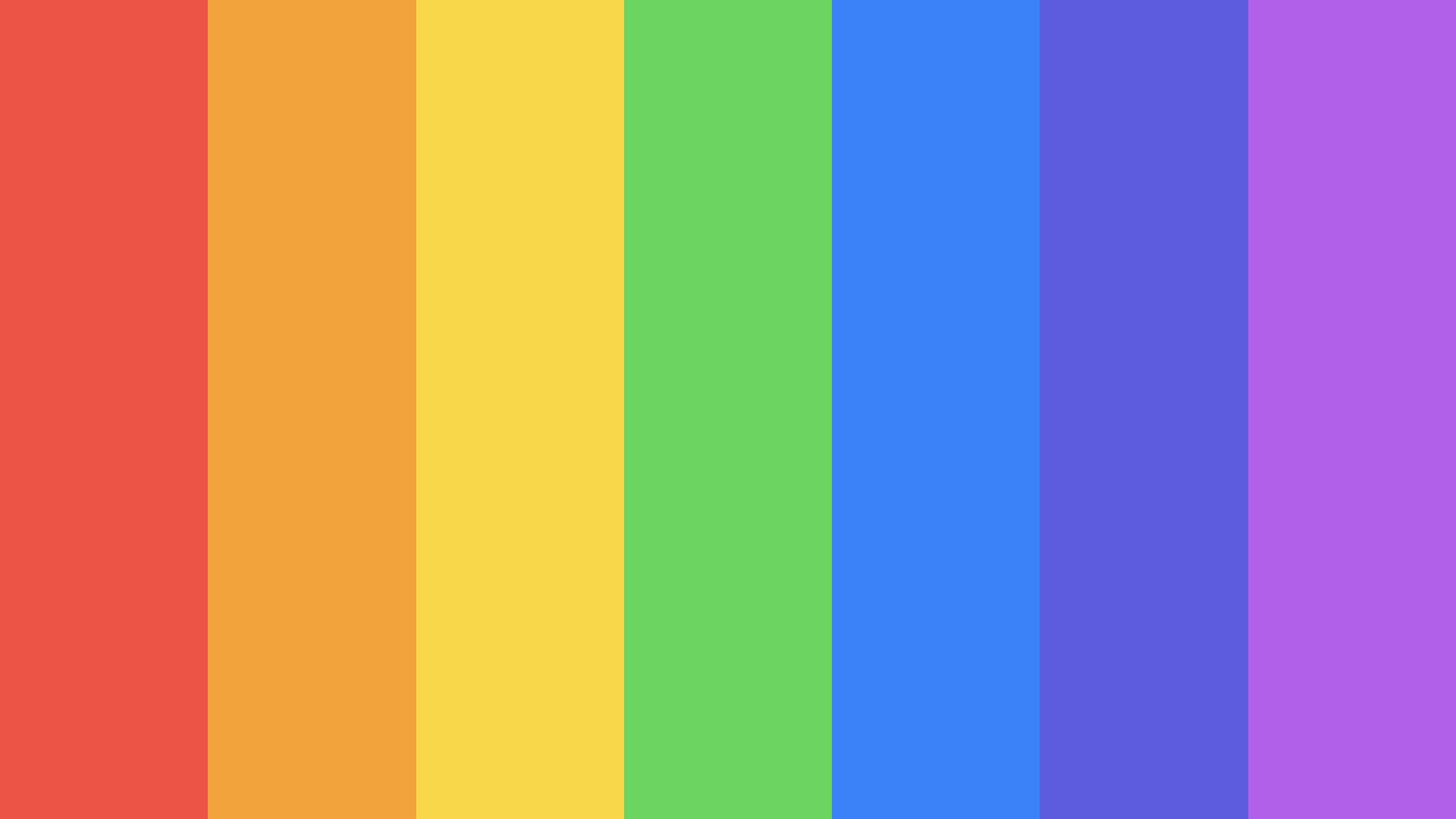
Colors from Component Values
You can create a custom color from component values like red, green, and blue values, or hue, saturation, and brightness values. These values should be supplied as a Double, between 0 and 1. For hue values, you may need to divide it by 360°, for red, green, and blue values, you may need to divide it by 255.
Note
Use Custom Colors using Asset Catalogue to adapt the color's value based on dark and light mode.
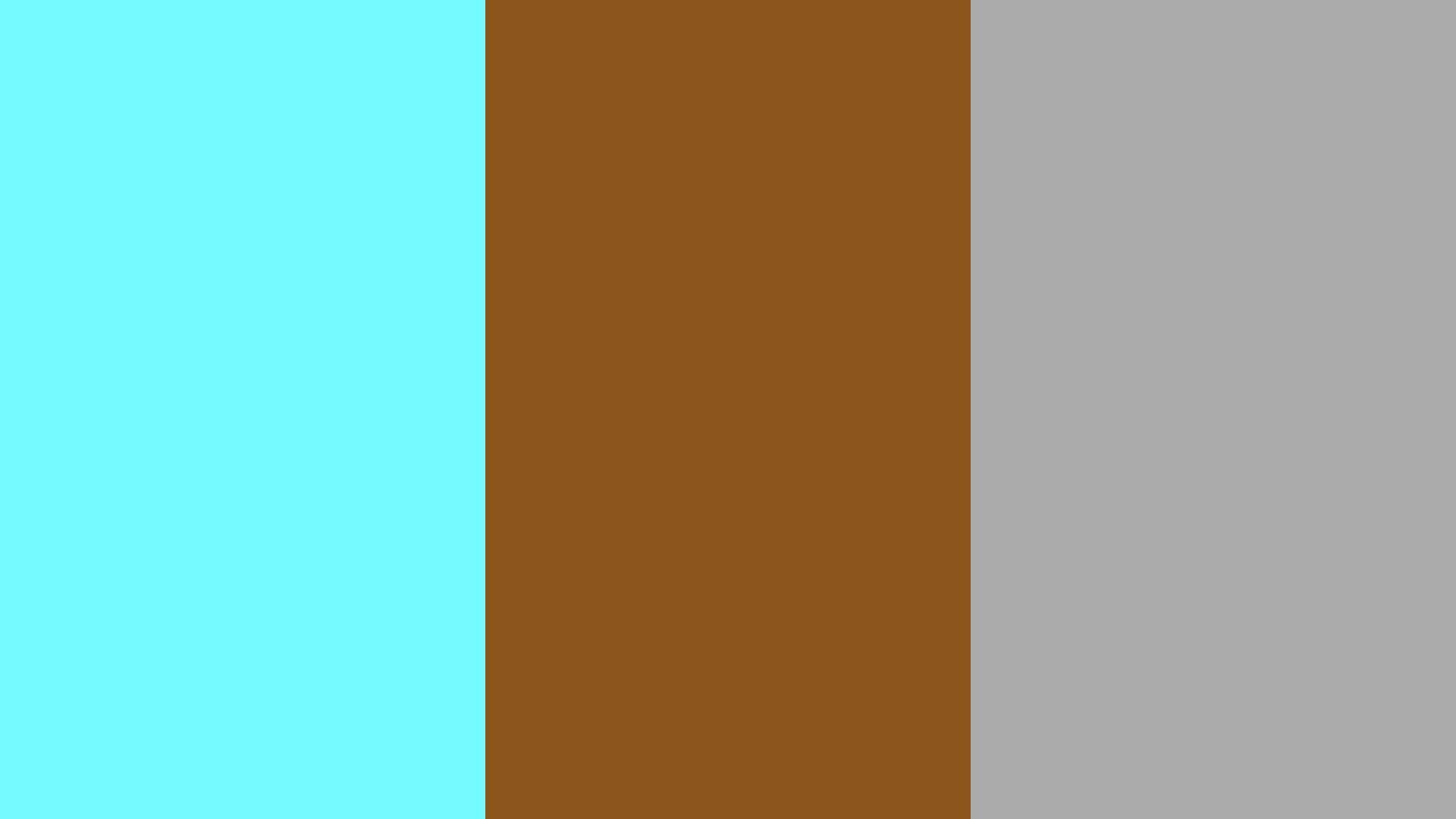
Colors as Views
In SwiftUI, Colors are also views. This allows you to manipulate them as you would a view.
In the following example, you can use SwiftUI colors to create the retro Apple logo with a VStack and a mask.
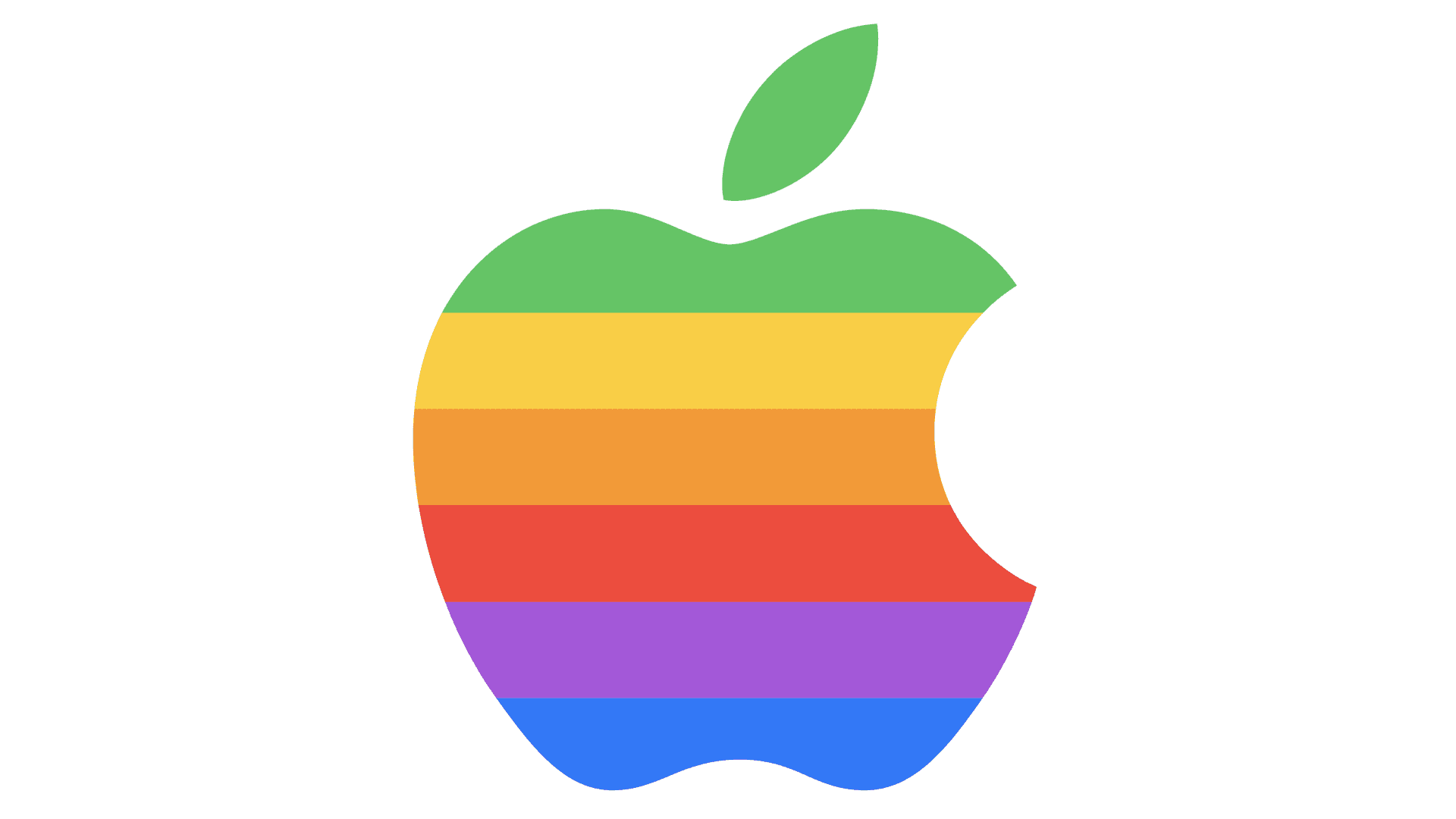
Background Colors
As colors fill as much space as they can (see Fitting & Filling Views), you can use a Color within a ZStack to set the background color of a View.
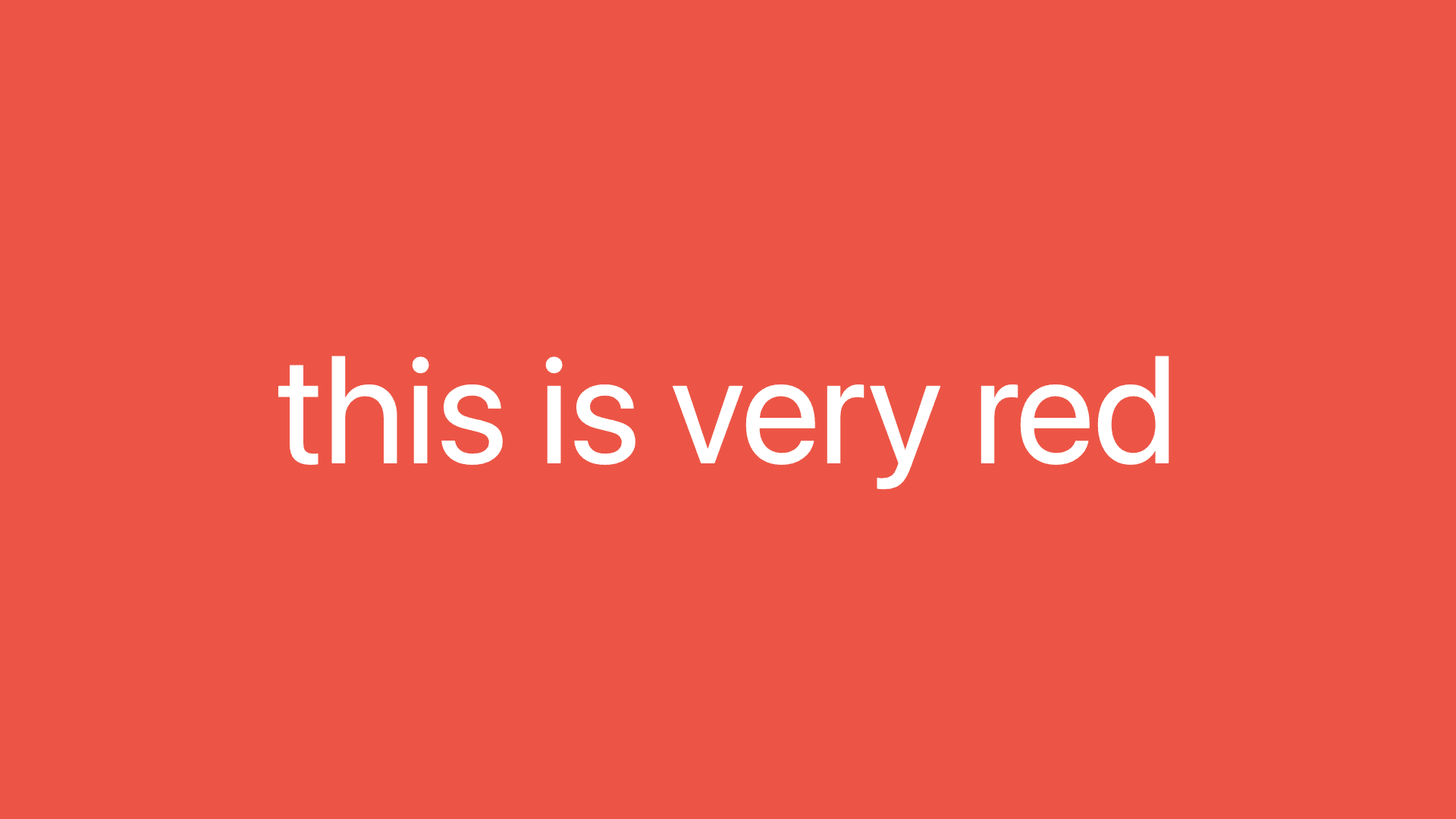
Colors as Variables
Colors are a unique type of view that can also function as a data store. This can be seen with a Color Picker storing a Color in a State variable.
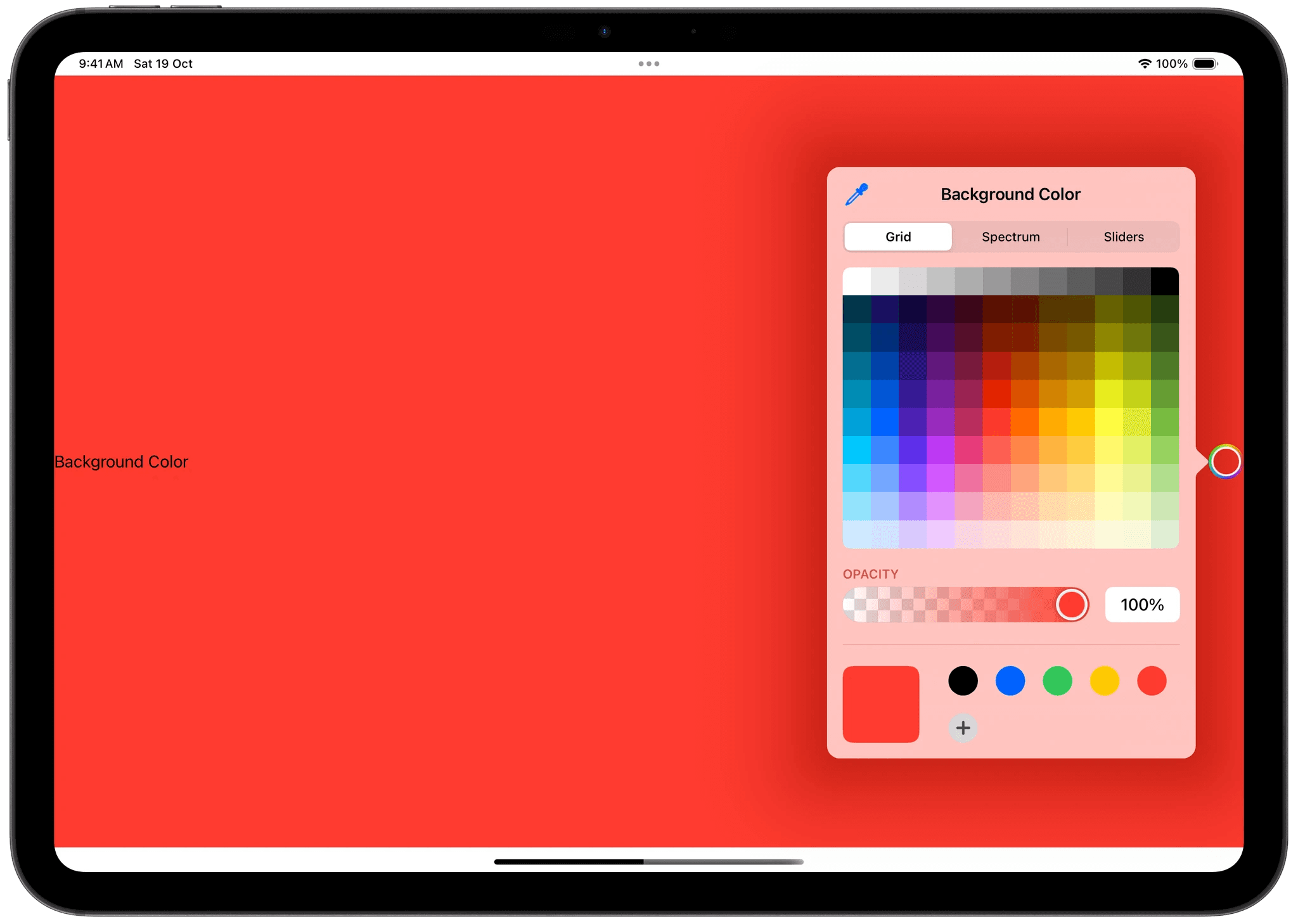
Custom Colors using Asset Catalog
Only available in Xcode, not supported in Swift Playground.
You can add Colors to the Asset Catalog with a Color Set.
From the Project Navigator, open the Assets Catalog
Click the + button at the bottom of the Assets Catalog
Choose Color Set
Rename the Color Set based on the color's purpose, like "BackgroundColor"
Select the color fields and customize it with the Attributes Inspector on the right, under the Color section.
By default, 2 color fields appear, Any Appearance and Dark.
Any Appearance is used for light mode, Dark is used for dark mode.
If you intend on choosing just one color, click on the entire section. From the Attributes Inspector, under Appearance, you can set it to None.
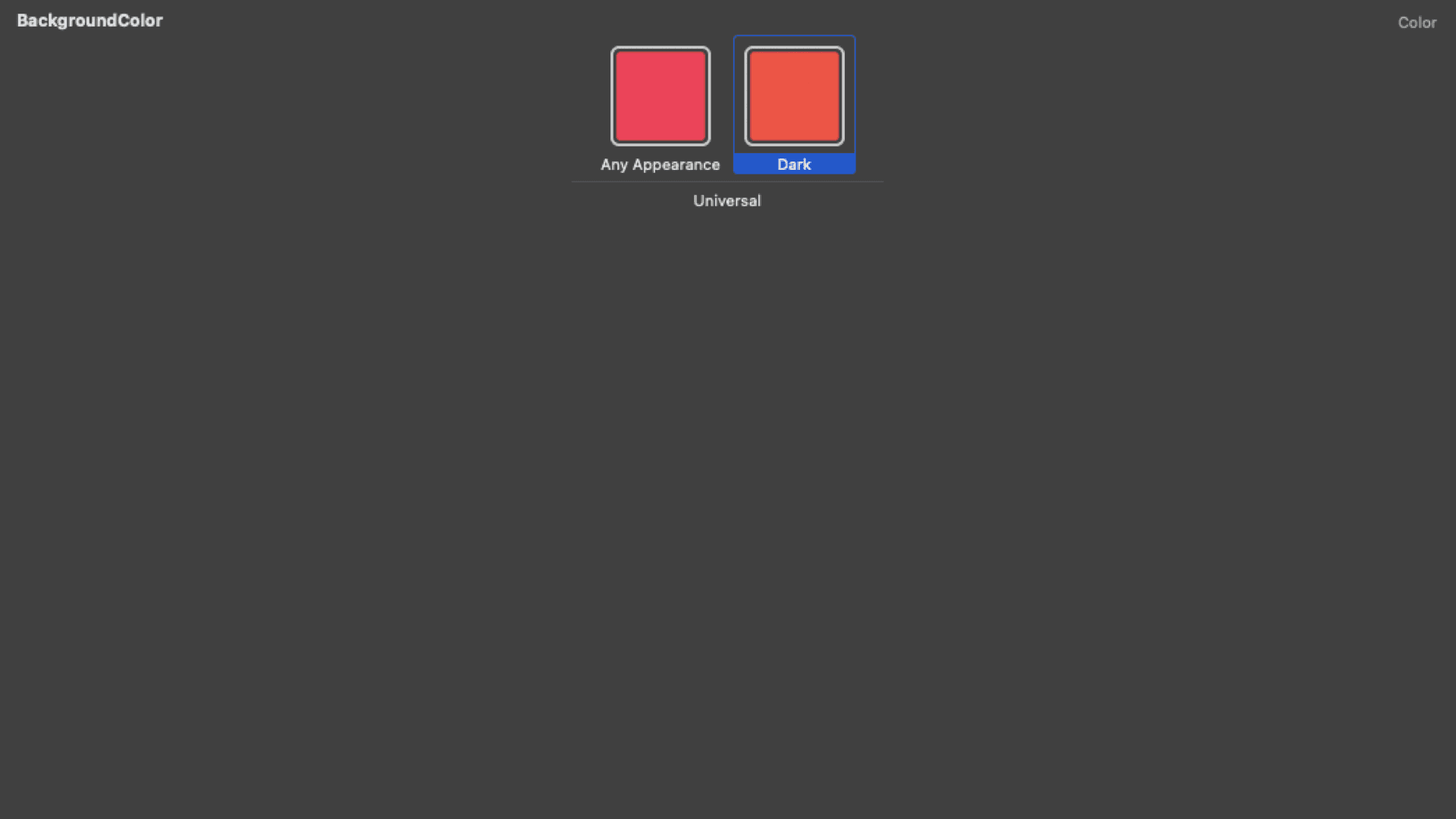
Using Custom Colors
You can use your custom colors in Swift by passing in the name of the color as a String.
Alternately, you can use the dot notation to retrieve your color. However, there are some things to note.
If your Color's name ends with "Color" in the asset catalog, the "Color" suffix will be omitted. The name will also be transformed into camelCase, like all variable names. "BackgroundColor" becomes "background"
Accent Color
Using the accent color is a great way to maintain consistency across your app's interface. Controls like Buttons and Text Field insertion points automatically update their appearance to fit the accent color.
Changing the Accent Color
In Swift Playground (iPad / Mac)
Open the sidebar
Select the App Settings
Set the Accent Color from the App Settings
In Swift Playground Project on Xcode
Open the Project Navigator
Choose the top-most file, the one with your project's name
Under App Settings, set the Accent Color.
In Xcode
Open the Assets Catalog
Select the AccentColor object in the Assets Catalog
Select the color field and customize the color in the Attributes Inspector
Using Accent Color
In SwiftUI, you can retrieve the accent color by using .accentColor
.
In These Collections
This article can also be found in these collections.