Video
Creating a Date Picker
At its minimum, a Date Picker has to be supplied with a label, to describe the purpose of the date picker, and a Binding date to be changed by the date picker.
In the example below, a birthday date picker is created with a "Birthday" label and a selection date.
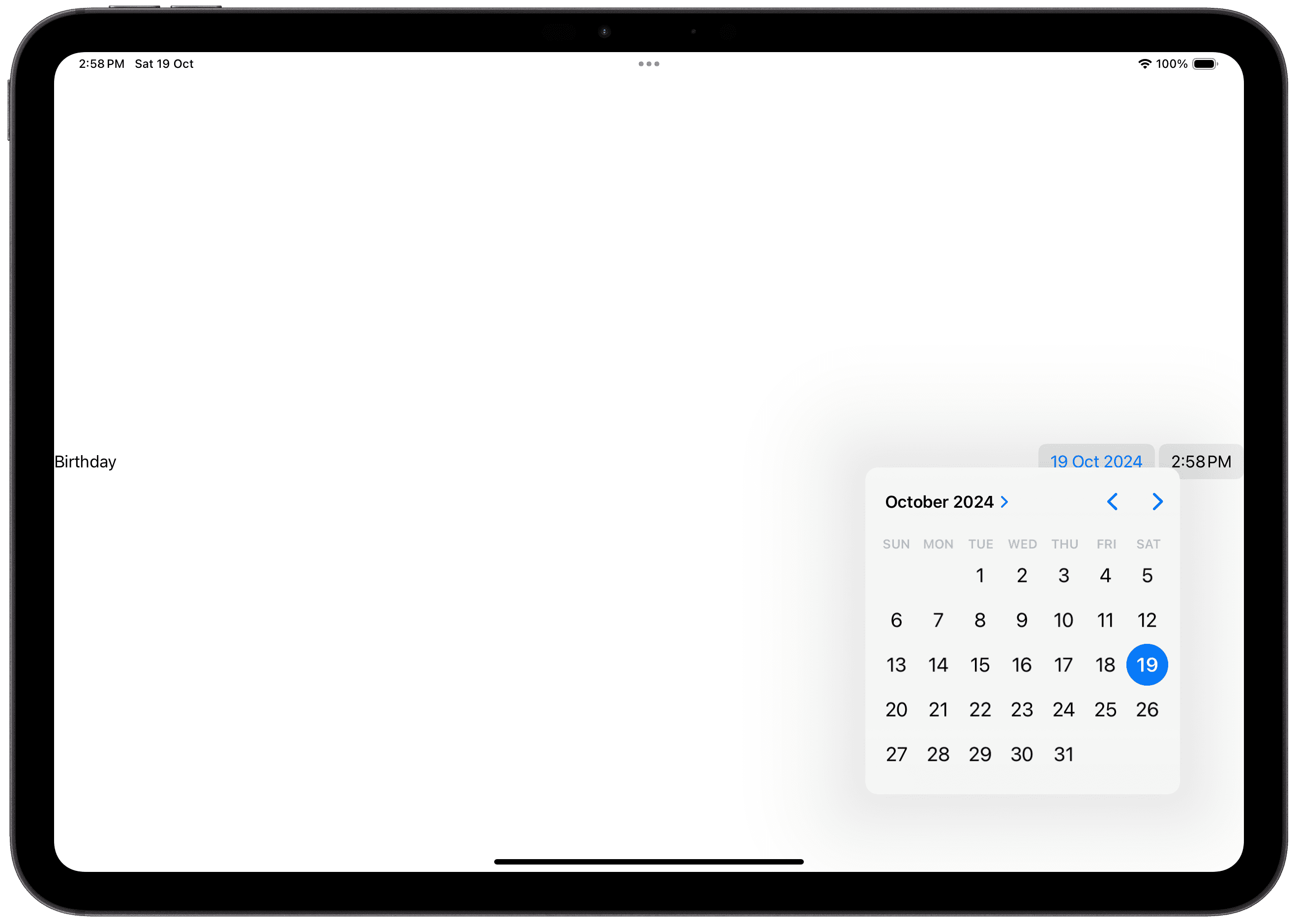
Basic Date Pickers
Text Label
Create a date State variable and assign it to the date picker with a Binding.
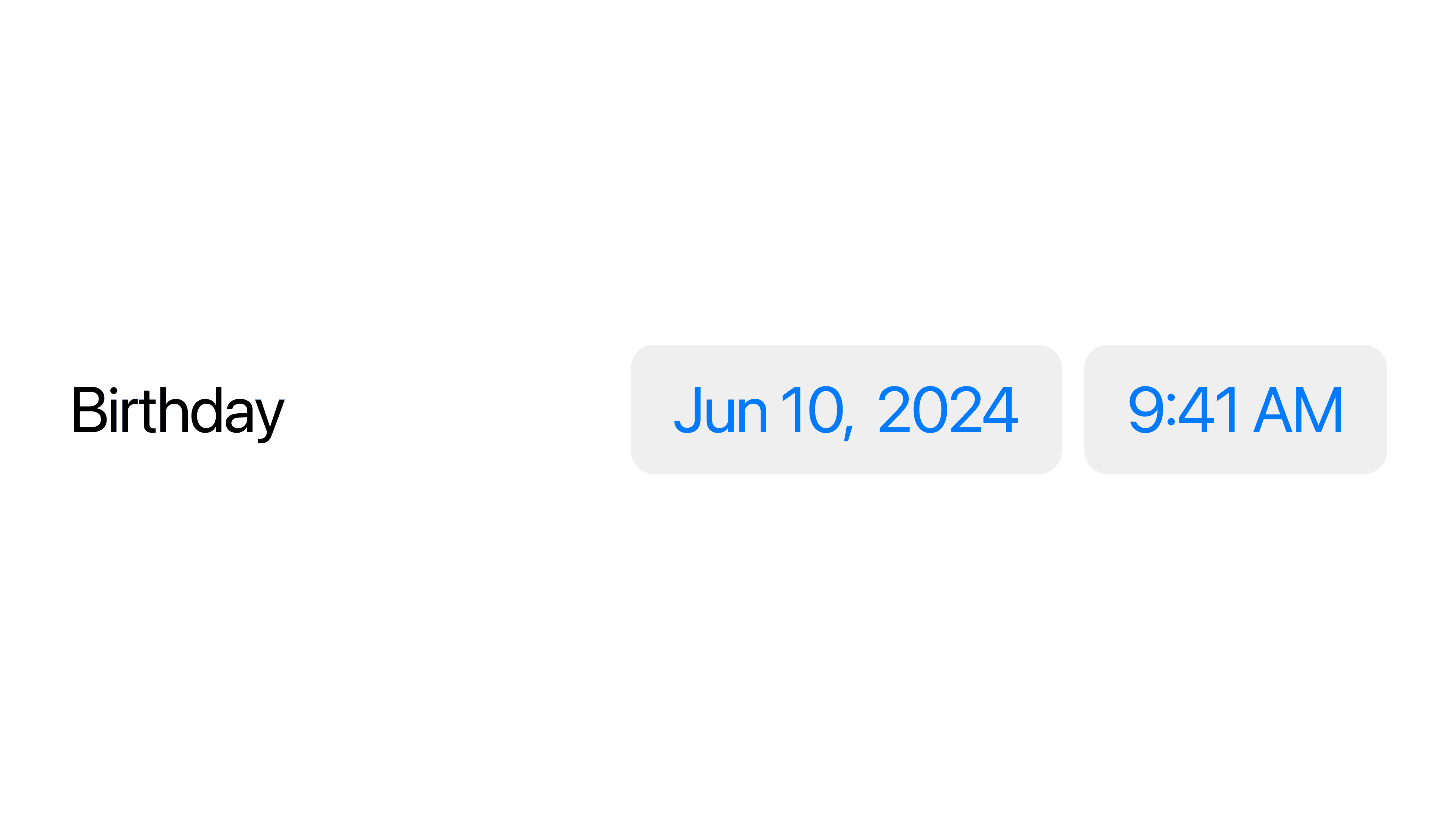
Custom Label
You can also pass in a custom view as the label.
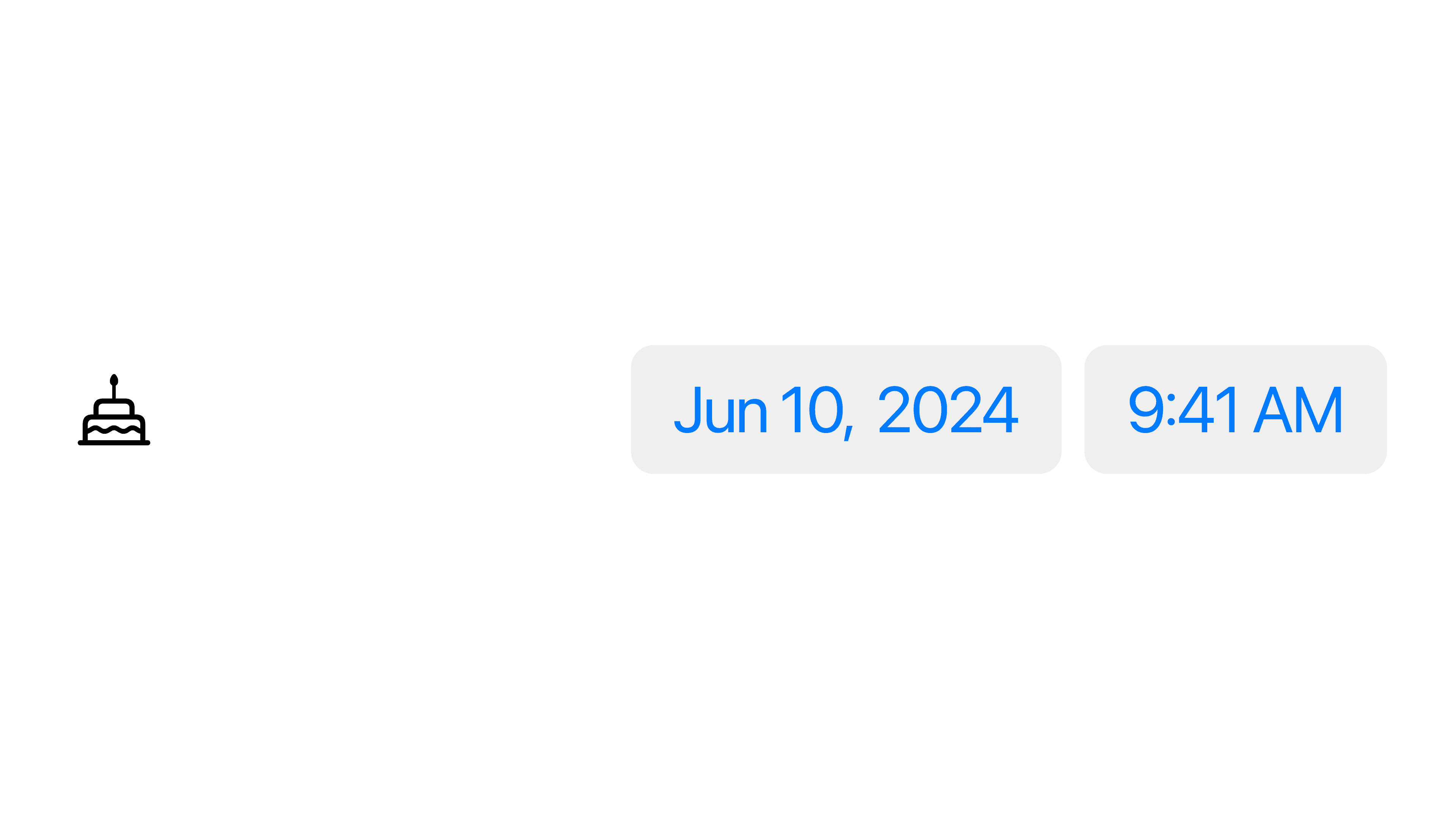
Displayed Components
You can customize what components appear in the date picker, the date, the time, or both.
To show just the date, use
displayedComponents: .date
To show just the time, use
displayedComponents: .hourAndMinute
To show both date and time, you can remove the
displayedComponents
parameter, or usedisplayedComponents: [.date, .hourAndMinute]
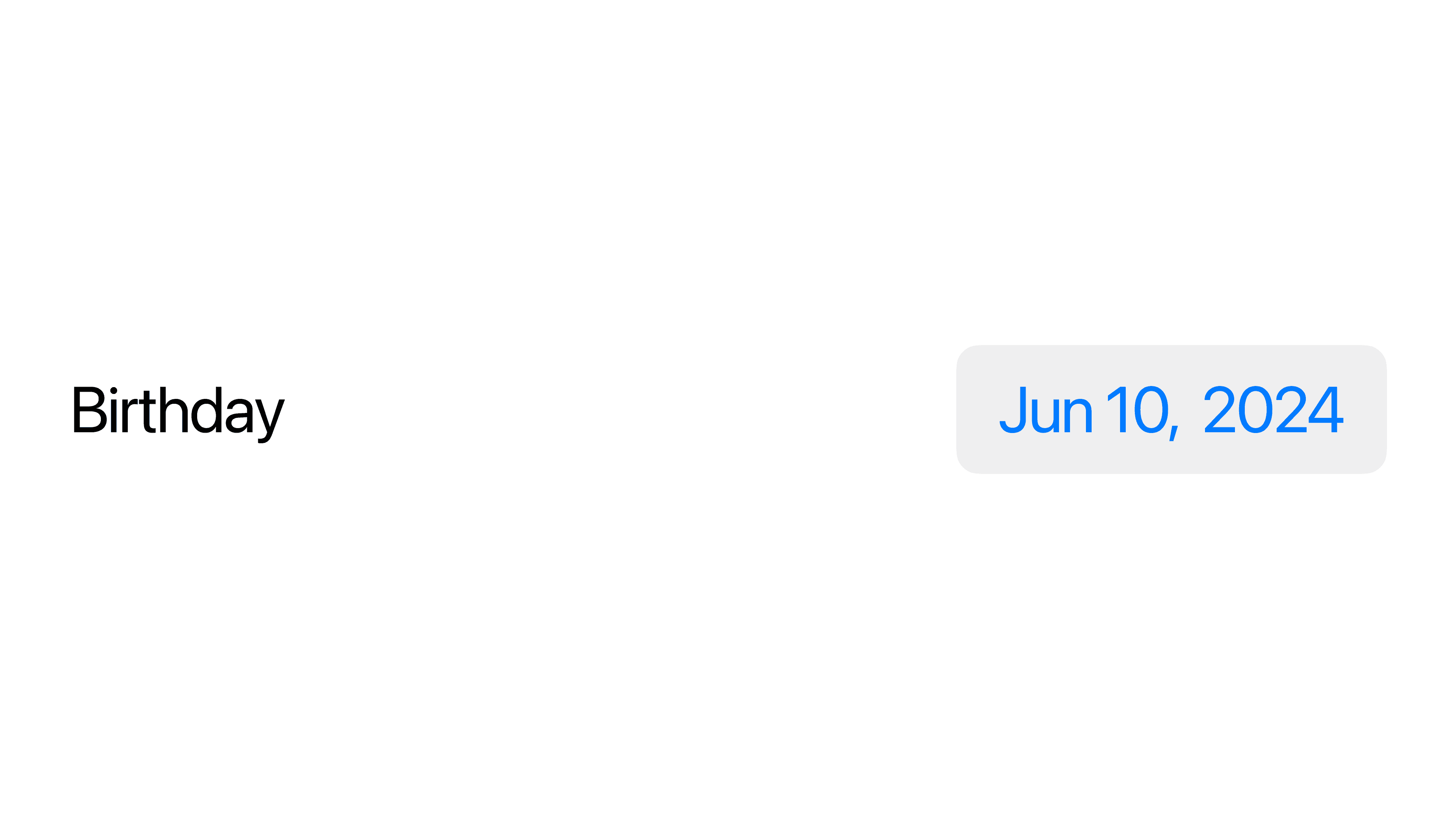
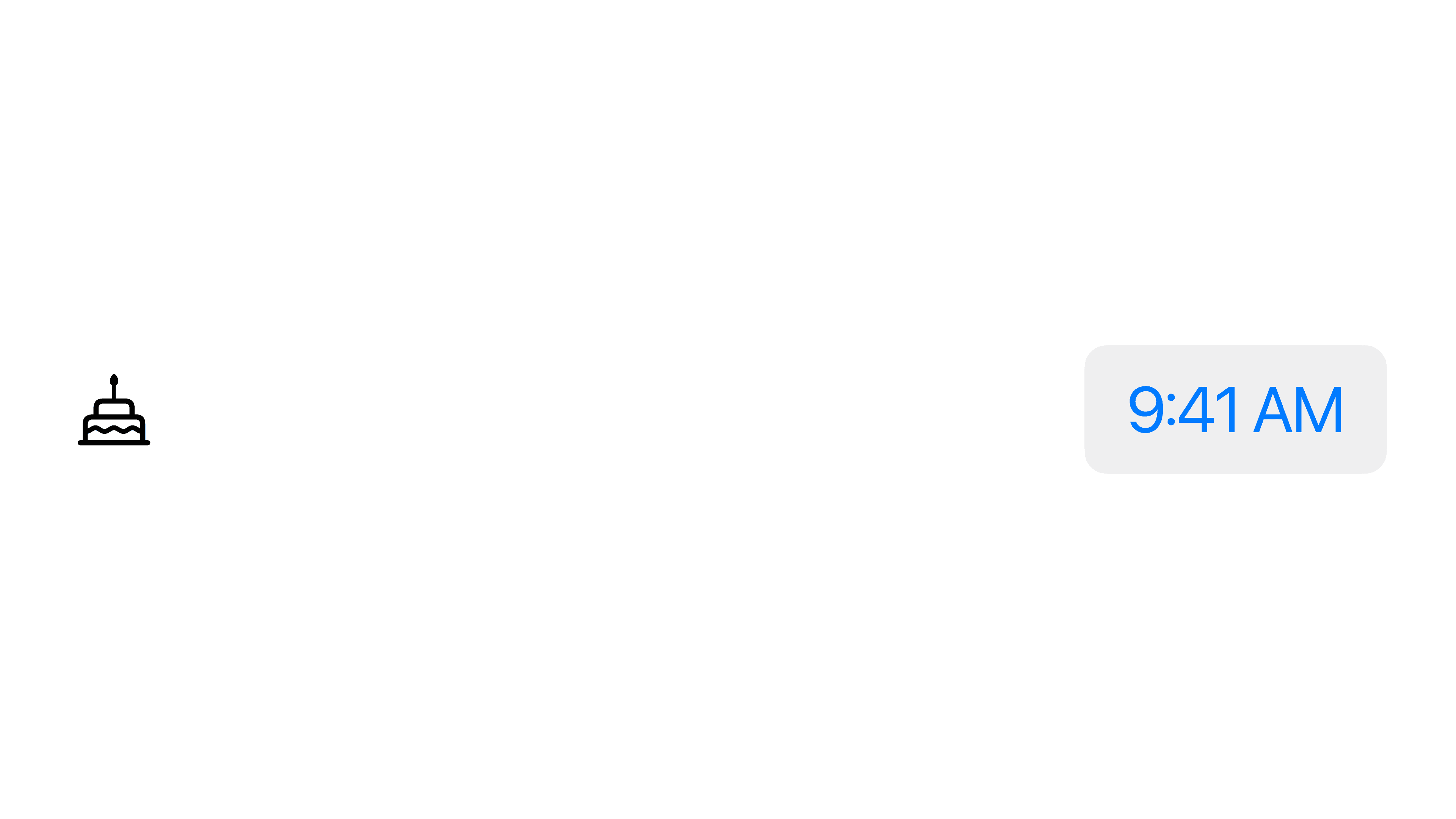
Date Ranges
You can customize the date picker's range to limit the valid inputs. In order to customize the range, you will need to supply it with a date range.
To create a custom date range with the
Date
type, usedate ... anotherDate
To restrict the user to selecting dates in the past, use
... Date.now
To restrict the user to selecting dates in the future, use
Date.now ...
In the following example, to create a date picker for a future event date, the Date.now ...
range is used to only allow the user to select dates in the future. In the date picker, unavailable dates are greyed out.
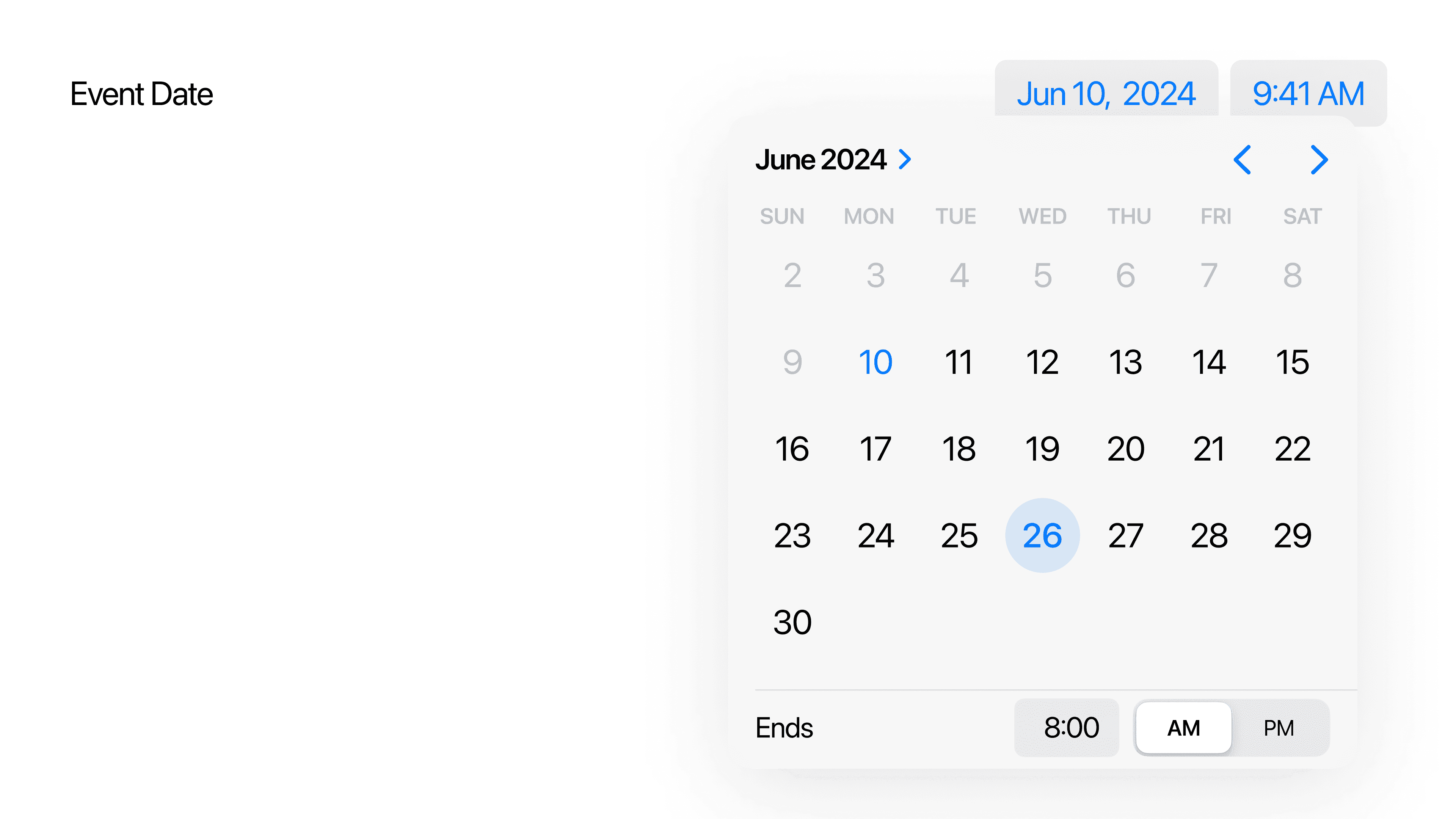
This also works with the custom label type.
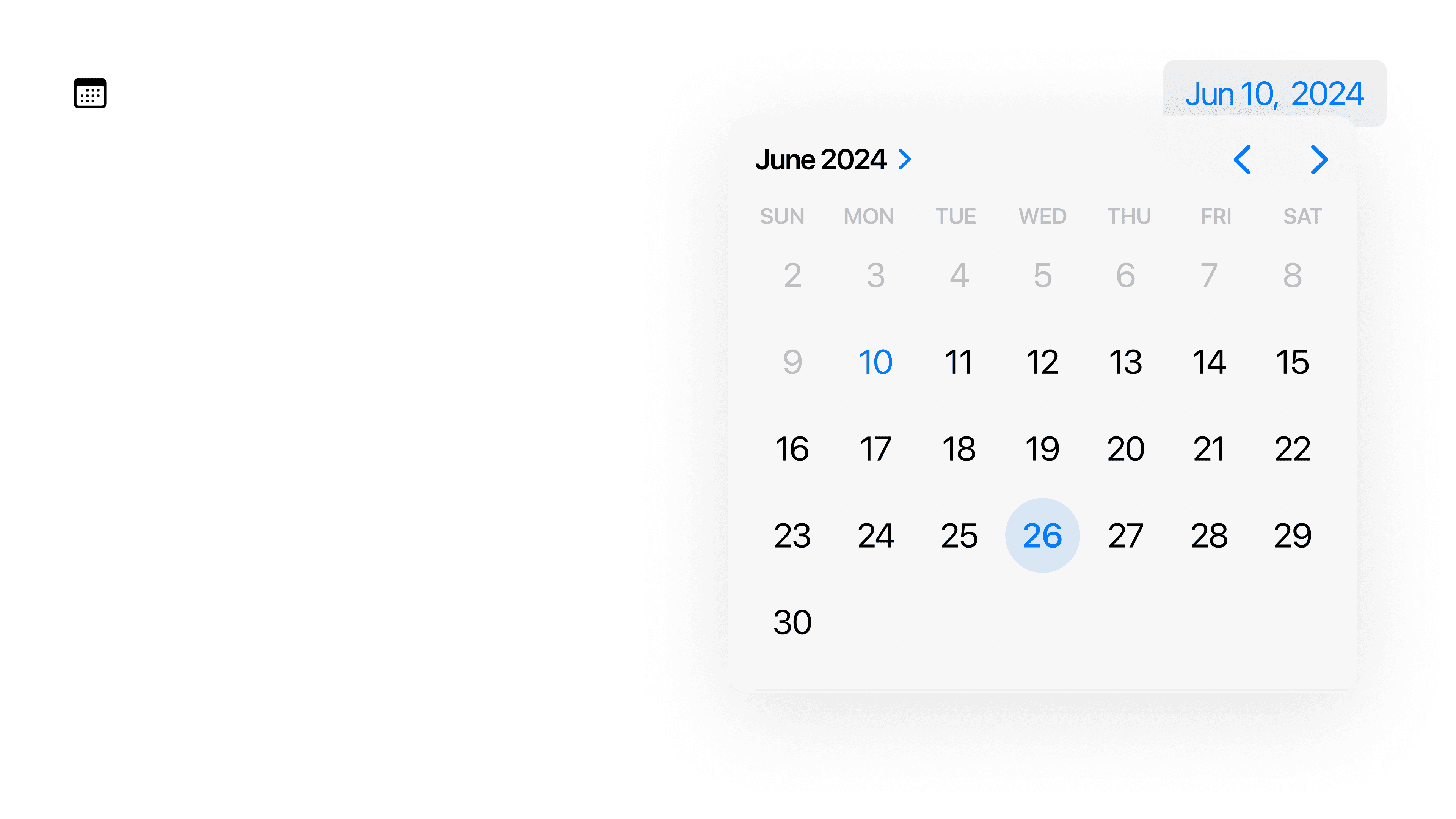
Date Ranges with Displayed Components
Date ranges also work with Displayed Components, allowing you to create a date picker to select specific timings, or one that selects just a specific date.
In this example, a date picker can be used to allow the user to select a time after the current time to book an appointment.
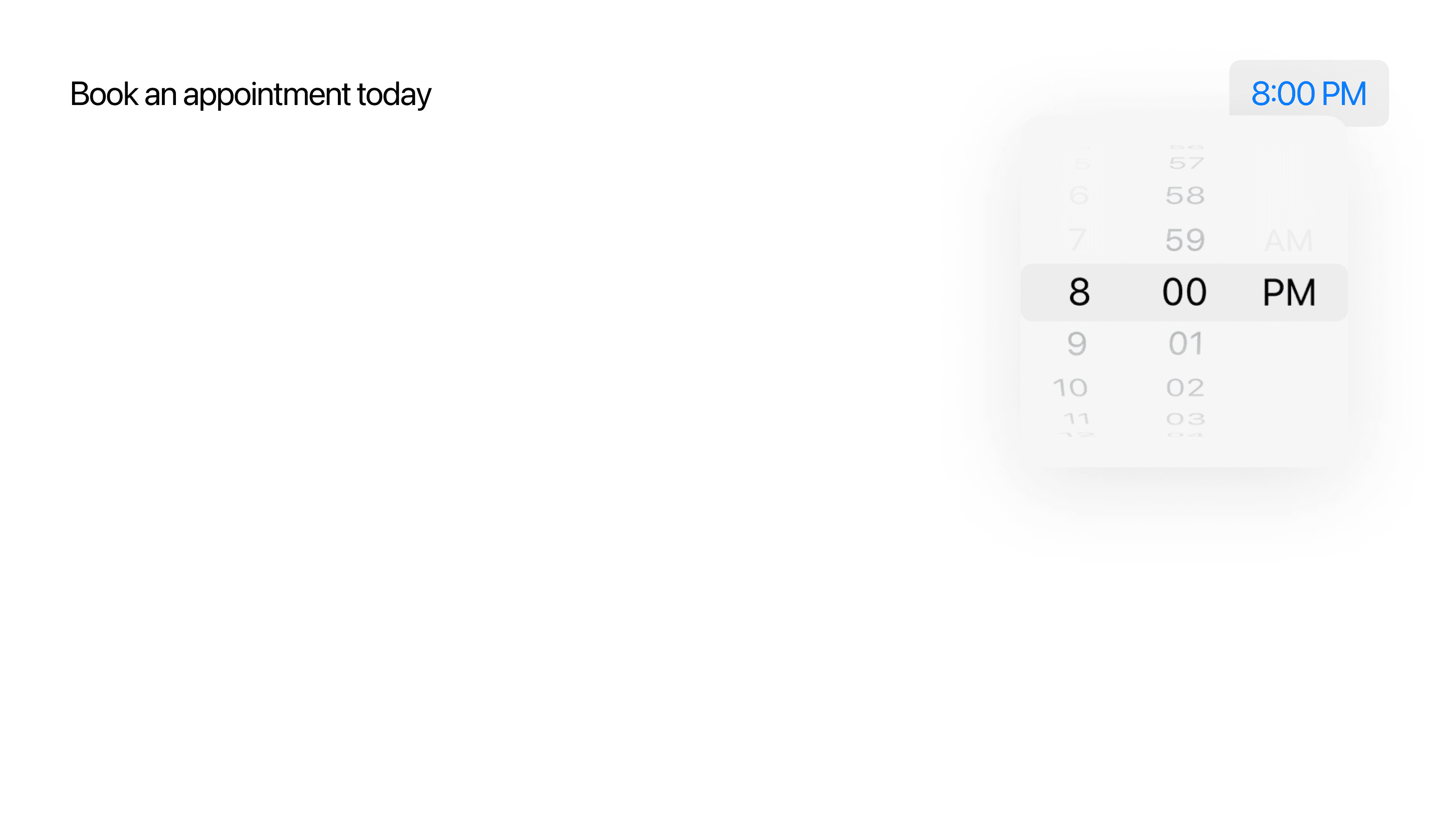
Similarly, this is also possible with custom labels.
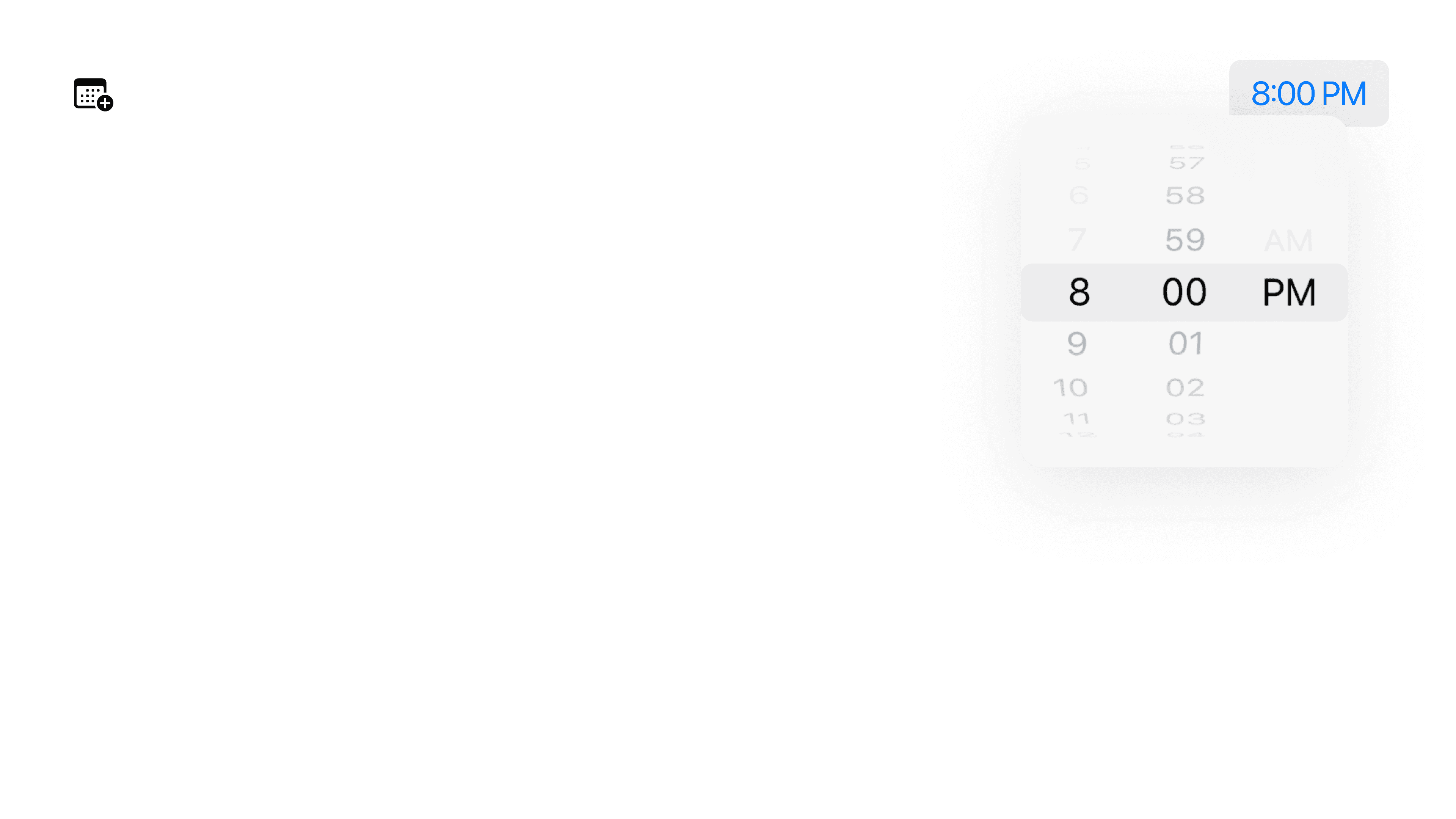
Styling Date Pickers
Like many other controls, date pickers also have a style modifier, datePickerStyle
. The styles are cascading, allowing you to apply it to a parent or ancestor view and have all date pickers adopt the style.
Compact
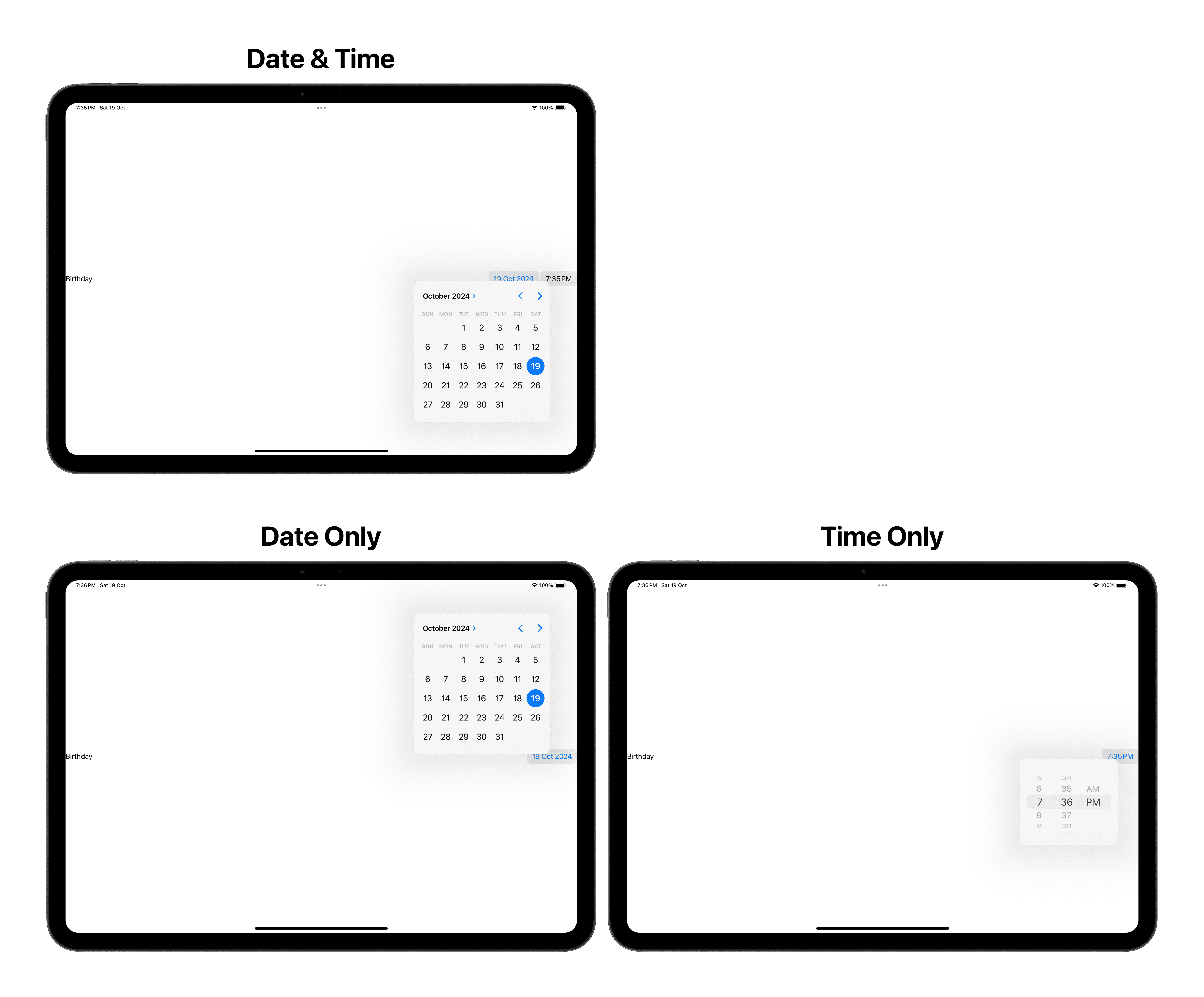
Graphical
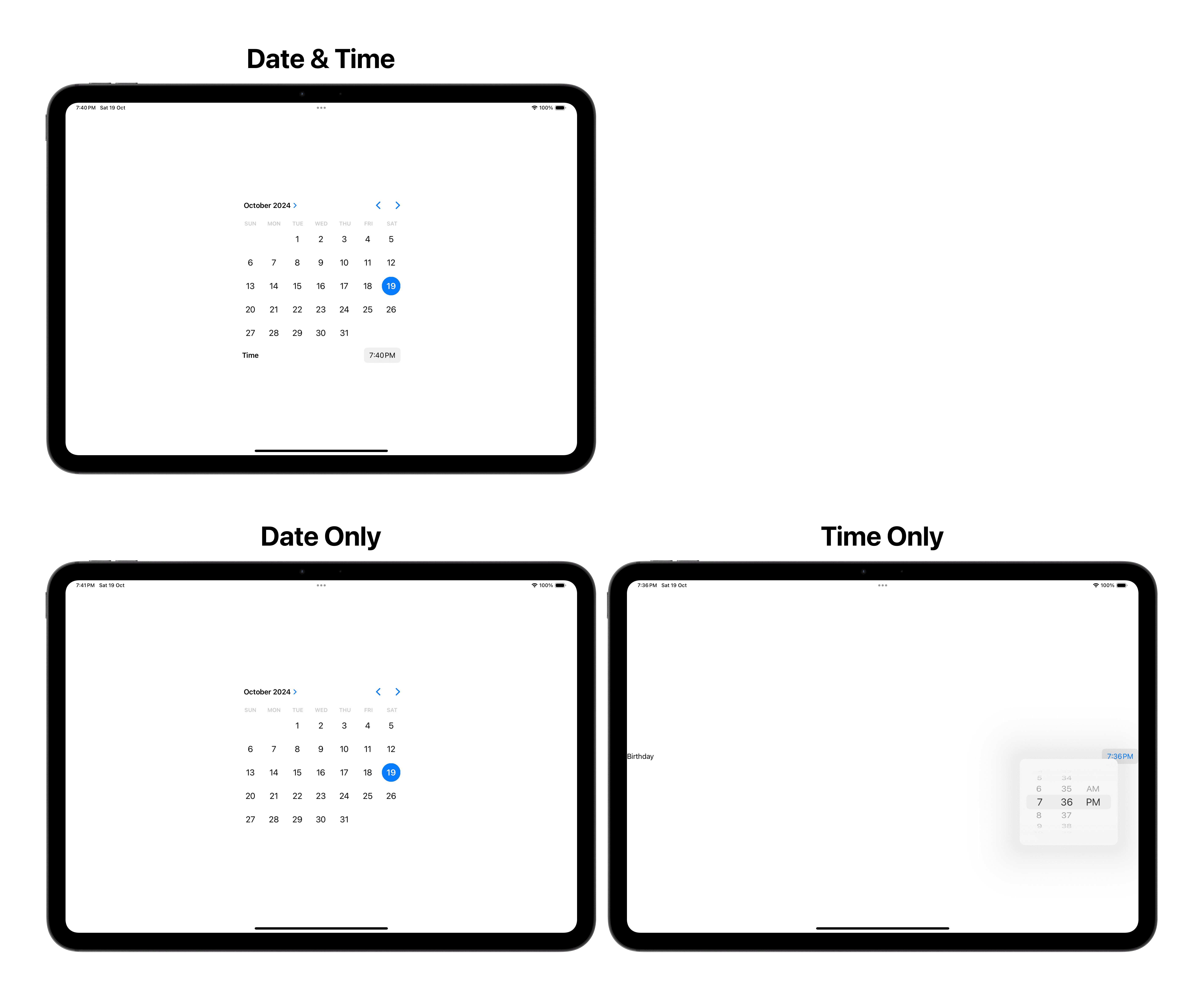
Wheel
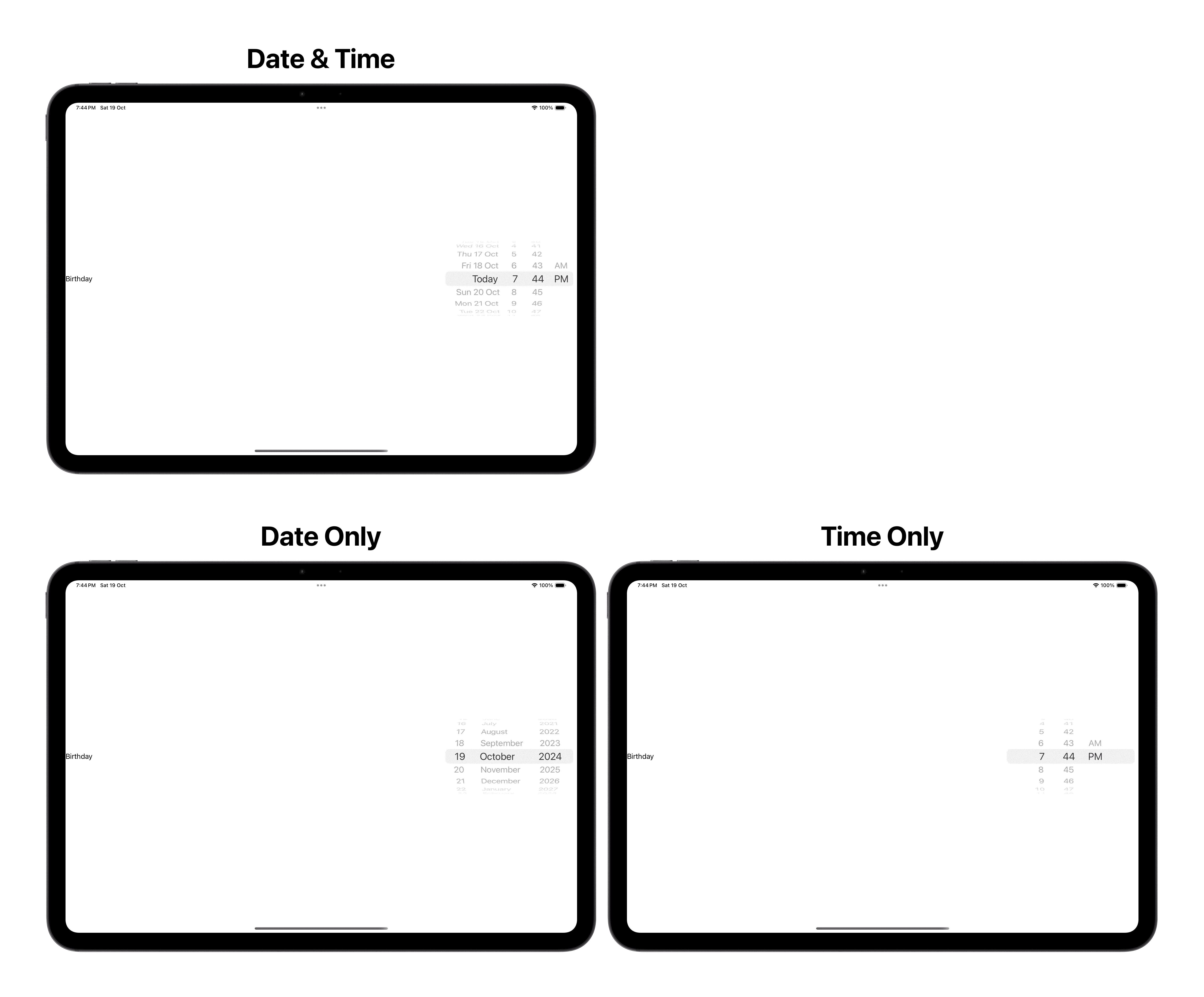
In These Collections
This article can also be found in these collections.